Published
- 11 min read
How to Scan Your Code for Vulnerabilities with GitHub Actions
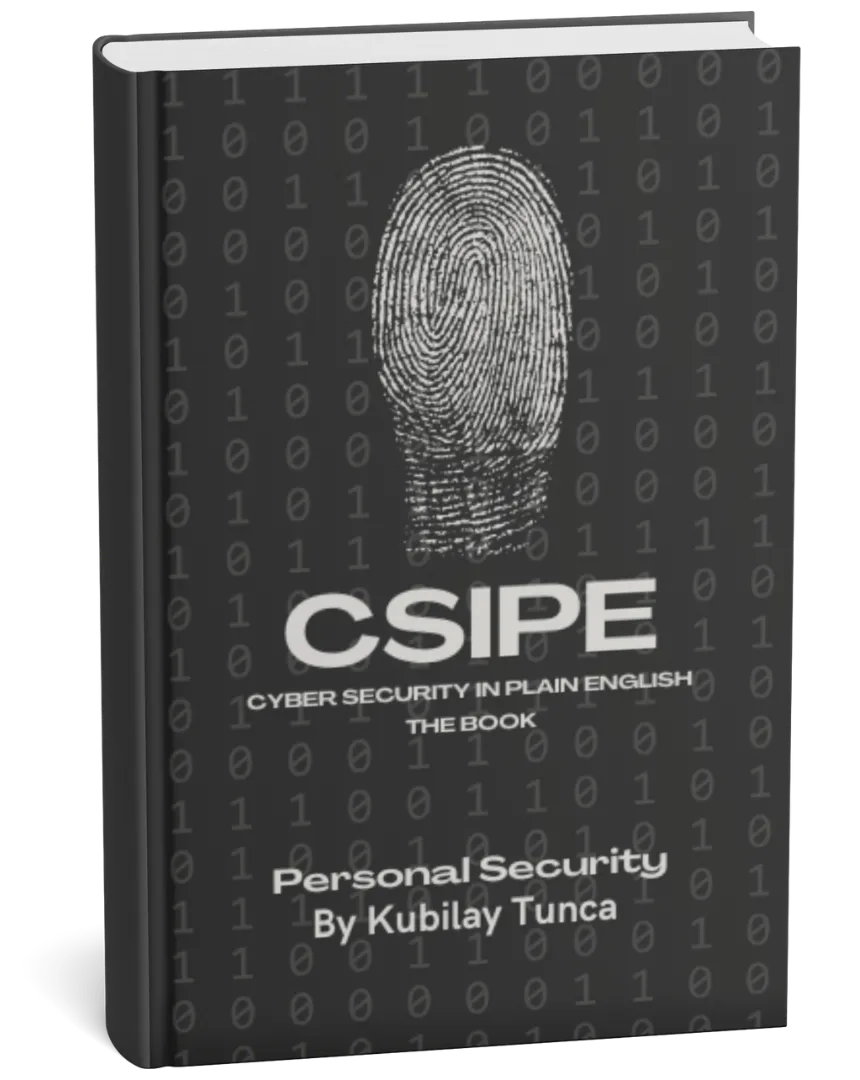
Protect Your Digital Life, Hassle-Free
Private Security in Plain English is your friendly guide to stopping common cyber threats—no tech degree required. Learn the simple, practical steps to safeguard your passwords, devices, and personal data in a language you’ll actually understand.
Buy the Ebook NowHow to Scan Your Code for Vulnerabilities with GitHub Actions
Security is no longer a luxury—it’s an integral requirement for modern software development. As developers, we carry the weight of ensuring our code not only runs efficiently but also stands firm against a never-ending stream of cyber threats. From overlooked SQL injections to the inadvertent use of outdated libraries, vulnerabilities can creep into any project. Fortunately, continuous integration and deployment (CI/CD) platforms now make it easier than ever to integrate security practices directly into your development workflow.
In this extensive guide, we’ll explore how to use GitHub Actions to automate the process of scanning your code for vulnerabilities. Whether you’re a solo developer maintaining a small open-source project or part of a large engineering team working on mission-critical applications, adding automated security scans can drastically improve your security posture. We’ll walk through the fundamentals, discuss popular scanning tools, explain best practices, and highlight patterns that keep your pipelines efficient and maintainable. By the end, you’ll have the knowledge you need to confidently incorporate vulnerability scanning into your CI/CD pipeline using GitHub Actions.
Table of Contents
- Dependency Vulnerabilities
- Code Quality and Insecure Coding Patterns
- Configuration and Infrastructure-as-Code Issues
- Secrets and Credential Leaks
- When to Run Security Scans
- Pull Request Checks vs. Scheduled Runs
- Failing the Build vs. Soft Reporting
- Setting Up a GitHub Actions Workflow File
- Integrating a Simple Vulnerability Scanner
- Analyzing Results and Reporting
- What is CodeQL?
- Configuring CodeQL in Your Workflow
- Interpreting CodeQL Results
- Custom Queries and Advanced Techniques
- Detecting Vulnerable Dependencies
- Software Bill of Materials (SBOM)
- Automating Dependency Updates with Dependabot
- Preventing Secrets from Entering the Repo
- Automated Secrets Scanning Tools
- Responding to Leaked Credentials
- Case Studies and Real-World Examples
- Future Trends and Emerging Best Practices
- Maintaining Your Security Action Workflows
Why Automate Security Scans?
Manual code reviews and periodic penetration tests are valuable, but in a fast-moving environment, they’re not enough. Developers frequently push updates, merge pull requests, and integrate new dependencies. Each new commit can introduce a potential flaw. By automating security scans, you ensure that every change is vetted before it reaches production. Automation helps:
- Catch vulnerabilities early: Identify issues when they’re cheap to fix.
- Enforce consistent standards: Ensure every code contribution meets a baseline level of security.
- Improve developer efficiency: Automated feedback reduces back-and-forth between security teams and developers.
- Facilitate compliance: Continuous scanning can assist with regulatory requirements and audits.
Understanding GitHub Actions
GitHub Actions provides a CI/CD solution baked right into the GitHub platform. With actions, you can trigger workflows based on events (like push
or pull_request
) and run jobs defined in YAML files right in your repository. For security scanning, this means no external servers or complex setups—just define your workflow, integrate a security tool, and you’re good to go.
Key Concepts of GitHub Actions
- Workflows: YAML files stored in
.github/workflows/
that define when and how your jobs run. - Jobs: Individual units of work performed by a runner. You can have multiple jobs that run in parallel or depend on each other.
- Steps: The commands and actions within a job. For security scanning, each step might install tools, run a scanner, and parse results.
- Actions: Pre-built or custom reusable steps. Many security tools provide their own GitHub Action for easy integration.
Pros and Cons for Security Workflows
Pros:
- Tight integration with GitHub repository events.
- Large ecosystem of pre-built actions for security scanning.
- Easy to manage and version alongside your code.
Cons:
- Running scans can consume CI minutes and resources.
- Complex or resource-intensive scans might require caching or optimization.
- Vendor lock-in if you rely heavily on GitHub-specific features.
Types of Vulnerabilities to Look For
Security isn’t just about SQL injections or cross-site scripting. Modern codebases have multiple layers and components, each with potential issues:
Dependency Vulnerabilities
Open-source libraries and frameworks can harbor known vulnerabilities. Attackers often target these known flaws. Keeping dependencies updated and scanned ensures you don’t ship known insecure versions.
Code Quality and Insecure Coding Patterns
Even if your dependencies are secure, your own code could have logic flaws, unvalidated inputs, or insecure cryptographic usage. Static analysis tools identify these patterns early.
Configuration and Infrastructure-as-Code Issues
Misconfigured cloud infrastructure, unsafe Terraform configurations, or exposed S3 buckets can be caught by scanning IaC templates or configuration files.
Secrets and Credential Leaks
Accidentally committing API keys or passwords can lead to immediate compromises. Automated scanners can detect and alert you to these leaks before they cause damage.
Choosing the Right Security Tools
Your choice of scanners depends on your language, codebase size, and security goals.
CodeQL
GitHub’s own CodeQL is a powerful semantic code analysis tool that finds subtle vulnerabilities by querying code semantics. It supports multiple languages and integrates seamlessly with GitHub Actions.
Semgrep
Semgrep offers customizable rule-based static analysis. It’s language-agnostic and has a large community of prebuilt rules, making it fast and flexible.
Trivy
Trivy scans containers, dependencies, and IaC for known issues. It’s excellent if you’re deploying containerized applications and need to check your images regularly.
Dependency Review Action
GitHub’s native Dependency Review action alerts you when pull requests introduce insecure dependencies. This is a quick win for preventing known vulnerabilities from entering your codebase.
Bandit, ESLint, and Other Linters
For Python, Bandit checks for common pitfalls. ESLint and security-oriented ESLint plugins protect JavaScript/TypeScript code. Specialized linters help you catch language-specific vulnerabilities early.
Planning Your GitHub Actions Workflows for Security
When to Run Security Scans
Common triggers include:
- On Pull Request: Ensure new code is secure before merging.
- On Push to Main: Validate that the main branch remains secure.
- Scheduled Runs: Periodic scans to catch newly discovered vulnerabilities in dependencies.
Pull Request Checks vs. Scheduled Runs
Pull Request Checks: Immediate feedback to developers, preventing vulnerable code from merging.
Scheduled Runs: Weekly or nightly scans can uncover vulnerabilities that appear after code is merged (e.g., newly reported CVEs in dependencies).
Failing the Build vs. Soft Reporting
Some teams choose to fail the build if a high-severity vulnerability is detected, preventing merges. Others only comment on the PR or send alerts, giving developers flexibility.
Implementing a Basic Security Workflow
Setting Up a GitHub Actions Workflow File
In .github/workflows/security-scan.yml
, define a basic workflow:
name: Security Scan
on: [pull_request, push]
jobs:
scan:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Run Security Scanner
run: |
# Example command for your chosen scanner
security-tool scan .
Integrating a Simple Vulnerability Scanner
If you pick a tool like Semgrep:
- name: Install Semgrep
run: pip install semgrep
- name: Run Semgrep
run: semgrep --config p/ci .
Analyzing Results and Reporting
Some scanners exit with a non-zero code if vulnerabilities are found. You can parse output and create comments on the PR. Or use built-in GitHub Actions to annotate code lines with warnings.
Using CodeQL for Deep Code Analysis
What is CodeQL?
CodeQL treats code as data and queries it for known vulnerability patterns. It’s powerful for complex codebases in languages like Java, JavaScript, Python, and C/C++.
Configuring CodeQL in Your Workflow
GitHub provides a starter template:
- name: Initialize CodeQL
uses: github/codeql-action/init@v2
- with:
- languages: javascript
- name: Perform CodeQL Analysis
- uses: github/codeql-action/analyze@v2
Interpreting CodeQL Results
CodeQL provides alerts in the Security tab of your GitHub repo. Each alert highlights the vulnerable code with remediation advice.
Custom Queries and Advanced Techniques
You can write custom CodeQL queries to catch organization-specific anti-patterns or compliance issues. Store these queries in your repo for consistent enforcement.
Integrating Semgrep for Faster, Customizable Checks
Installing Semgrep in a Workflow
- name: Install Semgrep
run: pip install semgrep
Configuring Rulesets
Semgrep allows you to specify rules via .semgrep.yml or directly reference community rules (semgrep —config p/r2c-ci).
Using Semgrep CI Integration
Semgrep provides a GitHub Action that directly posts findings as PR comments, making it even easier to integrate feedback into code reviews.
Dependency Scanning and SBOM Generation
Detecting Vulnerable Dependencies
Tools like npm audit, yarn audit, or pip-audit can run within GitHub Actions. For example:
- name: NPM Audit
run: npm audit --json
If vulnerabilities exist, you can fail the build or create PR comments.
Software Bill of Materials (SBOM)
Generate an SBOM with tools like cyclonedx-cli. Storing an SBOM helps track what dependencies you have and if they become vulnerable in the future.
Automating Dependency Updates with Dependabot
Enable Dependabot to open PRs updating dependencies. Combine this with scanning actions to ensure updates are secure.
Container and Infrastructure Scanning
Scanning Docker Images with Trivy
- name: Scan Docker Image with Trivy
run: trivy image --exit-code 1 myimage:latest
If vulnerabilities are found, Trivy returns a non-zero exit code, failing the job.
Checking IaC Files with Terraform Scanners
Use IaC security tools like tfsec or checkov to detect misconfigurations in Terraform, Kubernetes manifests, or CloudFormation templates.
Secrets Detection and Credential Hygiene
Preventing Secrets from Entering the Repo
GitHub’s built-in secret scanning can alert you if credentials slip into your code. Integrate tools like gitleaks in your workflow.
- name: Gitleaks
run: gitleaks detect --exit-code 1
Automated Secrets Scanning Tools
Use actions that specifically scan for secrets, such as github/codeql-action with secret scanning or third-party tools that comment on PRs.
Responding to Leaked Credentials
If keys are found, rotate them immediately, remove them from the repo’s history, and add safeguards to prevent future occurrences (like commit hooks and pre-commit checks).
Optimizing Performance and Reducing Noise
Caching and Parallelization
Use GitHub Actions caching to speed up dependency installs. Run scans in parallel to reduce total CI time.
Selective Runs Based on Changed Files
Trigger scans only if certain directories change. For example, run IaC scans only if .tf files changed. This reduces unnecessary scans and speeds up feedback.
Suppressing False Positives
All scanners can produce false positives. Configure rules to ignore known patterns or whitelist certain code constructs. Maintain a .semgrepignore or .codeqlignore file.
Collaboration and Reporting
Pull Request Comments and Status Checks
Automatically comment on PRs with detected vulnerabilities. Use GitHub’s checks API to provide a pass/fail status. This ensures developers see issues right where they’re most relevant—in the code review.
Slack and Email Notifications
Integrate with Slack or email for critical alerts. If a severe vulnerability is found, notify the security team immediately.
Dashboards and Metrics
Build dashboards in tools like Grafana or use GitHub’s Security tab to track trends over time. Are you reducing high-severity flaws? Are new vulnerabilities introduced at a lower rate?
Compliance, Governance, and Audit Trails
Documenting Your Security Pipelines
Write detailed README files or docs explaining which tools run, when they run, and what policies they enforce. Good documentation makes onboarding new developers easier and supports audits.
Audit Logging and Artifact Storage
Store logs and reports as artifacts. For compliance, you might need to prove that scans occurred and show the results over time.
Case Studies and Real-World Examples
-
Open Source Projects: Many OSS maintainers run CodeQL or Semgrep on PRs, ensuring community contributions are safe.
-
Enterprise Teams: Large organizations often integrate CodeQL with custom queries to enforce corporate security standards. They run nightly scans on main branches and provide dashboards to leadership.
-
Startup Environments: Startups use lightweight tools like Semgrep to quickly catch mistakes, balancing speed with security.
Future Trends and Emerging Best Practices
-
Integration with SAST/DAST Tools: Expect deeper integrations as more security vendors provide GitHub Actions.
-
Shift-Left Security: More scanning rules will be developer-friendly and language-agnostic, empowering devs to fix issues quickly.
-
Machine Learning and Heuristics: Future scanners may use ML to reduce false positives and understand code context better.
Maintaining Your Security Action Workflows
Versioning and Updating Scanners
Regularly update your actions and scanners to benefit from performance improvements and new vulnerability signatures.
Periodic Rule and Policy Reviews
As your codebase evolves, refine your scanning rules. Remove outdated checks, add new rules for modern frameworks, and respond to developer feedback on noise levels.
Conclusion
Integrating automated vulnerability scanning with GitHub Actions transforms your CI/CD pipeline into a robust defense mechanism. By scanning on pull requests, pushes, or on a schedule, you catch vulnerabilities before they harm your users or reputation. Whether you choose CodeQL for deep semantic analysis or Semgrep for rapid rule-based checks, the integration process is straightforward, and the payoff is substantial.
Key Takeaways:
-
Proactive Security: Automated scans mean you don’t rely solely on occasional manual reviews.
-
Developer-Friendly: Seamless integration with GitHub ensures developers receive immediate feedback.
-
Scalability: As your codebase grows, your security scans grow with it, maintaining a consistent standard.
By employing these strategies, tools, and best practices, you’ll create a sustainable, secure development environment. Over time, you’ll see fewer vulnerabilities making it to production, more confidence in your code quality, and a more streamlined collaboration between developers and security teams. In an age where security breaches are front-page news, this proactive approach is both a necessity and a competitive advantage.