Published
- 3 min read
How SSL/TLS Works: A Developer’s Guide
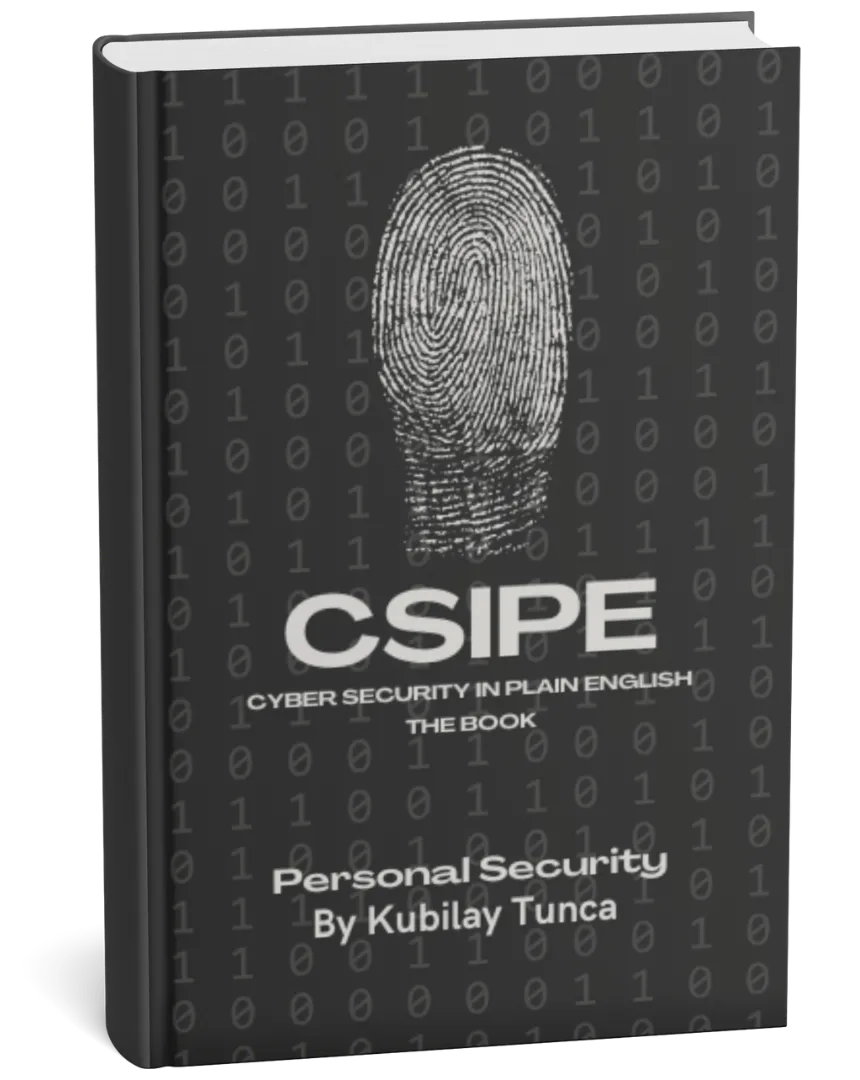
Protect Your Digital Life, Hassle-Free
Private Security in Plain English is your friendly guide to stopping common cyber threats—no tech degree required. Learn the simple, practical steps to safeguard your passwords, devices, and personal data in a language you’ll actually understand.
Buy the Ebook NowIntroduction
SSL (Secure Sockets Layer) and TLS (Transport Layer Security) are cryptographic protocols that ensure secure communication over the internet. They are foundational to modern web security, encrypting data to protect it from interception and tampering. For developers, understanding how SSL/TLS works and implementing it correctly is critical for safeguarding applications and their users.
This guide breaks down the mechanics of SSL/TLS, its components, and how to implement it effectively in your projects.
What is SSL/TLS?
SSL/TLS protocols secure data transmitted over a network by encrypting it, ensuring confidentiality, integrity, and authenticity. While SSL is the predecessor of TLS, TLS is now the standard due to its improved security features.
Key Goals of SSL/TLS:
- Encryption: Ensures that data is only readable by intended recipients.
- Authentication: Verifies the identity of communicating parties using certificates.
- Integrity: Prevents tampering with transmitted data.
How SSL/TLS Works
SSL/TLS relies on a handshake process to establish a secure connection. Here’s an overview of the steps:
1. Client Hello
The client initiates the handshake by sending a “Client Hello” message, including supported encryption algorithms and protocol versions.
2. Server Hello
The server responds with a “Server Hello,” selecting the encryption algorithm and sending its digital certificate.
3. Key Exchange
The client verifies the server’s certificate and generates a session key, which is securely shared with the server.
4. Secure Communication
The session key is used to encrypt subsequent communications between the client and server.
Key Components of SSL/TLS
1. Certificates
Digital certificates, issued by Certificate Authorities (CAs), verify the identity of a server or client.
Example (Generating a Self-Signed Certificate with OpenSSL):
openssl req -x509 -nodes -days 365 -newkey rsa:2048 -keyout server.key -out server.crt
2. Public and Private Keys
- Public Key: Encrypts data during the handshake.
- Private Key: Decrypts the data on the server side.
3. Cipher Suites
Cipher suites define the algorithms used for encryption, key exchange, and message authentication.
Example (Common Cipher Suite):
TLS_ECDHE_RSA_WITH_AES_256_GCM_SHA384
4. Protocol Versions
- TLS 1.2: Widely used and secure.
- TLS 1.3: The latest version with improved performance and security.
Implementing SSL/TLS in Applications
1. Using HTTPS for Web Applications
Example (Node.js with HTTPS Module):
const https = require('https')
const fs = require('fs')
const options = {
key: fs.readFileSync('server.key'),
cert: fs.readFileSync('server.crt')
}
https
.createServer(options, (req, res) => {
res.writeHead(200)
res.end('Secure Connection Established')
})
.listen(443)
2. Securing APIs
Use HTTPS for API endpoints to ensure encrypted communication.
Example (Express.js Middleware):
const express = require('express')
const app = express()
app.use((req, res, next) => {
if (!req.secure) {
return res.redirect(`https://${req.headers.host}${req.url}`)
}
next()
})
3. Configuring TLS for Databases
Ensure database connections use TLS for data encryption.
Example (PostgreSQL Connection):
psql "sslmode=require host=example.com dbname=mydb user=myuser"
Best Practices for SSL/TLS
1. Use Strong Cipher Suites
Configure your servers to use strong encryption algorithms and disable weak ciphers.
Example (Nginx TLS Configuration):
ssl_protocols TLSv1.2 TLSv1.3;
ssl_ciphers HIGH:!aNULL:!MD5;
2. Regularly Renew Certificates
Keep certificates up to date to avoid expiration-related disruptions.
3. Enable HSTS
HTTP Strict Transport Security (HSTS) ensures browsers always use HTTPS.
Example (Nginx HSTS Header):
add_header Strict-Transport-Security "max-age=31536000; includeSubDomains" always;
4. Perform Vulnerability Scans
Use tools like SSL Labs to analyze and improve your server’s SSL/TLS configuration.
5. Monitor for Certificate Revocation
Regularly check certificate status using Online Certificate Status Protocol (OCSP) or Certificate Revocation Lists (CRLs).
Common Challenges and Solutions
Challenge: Certificate Management
Solution:
- Use automation tools like Certbot to manage certificates.
Challenge: Compatibility Issues
Solution:
- Test SSL/TLS configurations across browsers and devices to ensure compatibility.
Challenge: Performance Overhead
Solution:
- Use TLS 1.3 for faster handshakes and optimized performance.
Tools for SSL/TLS
1. Certbot
Automates the process of obtaining and renewing SSL certificates from Let’s Encrypt.
2. OpenSSL
A toolkit for SSL/TLS implementation and testing.
3. SSL Labs
Provides detailed analysis and grading of your server’s SSL/TLS setup.
Conclusion
SSL/TLS is indispensable for securing data transmission in modern applications. By understanding its workings and following best practices, developers can create secure, reliable communication channels that protect users and applications alike.
Start implementing SSL/TLS in your projects today to ensure robust security and build trust with your users.