Published
- 4 min read
Implementing Secure Defaults in Popular Frameworks
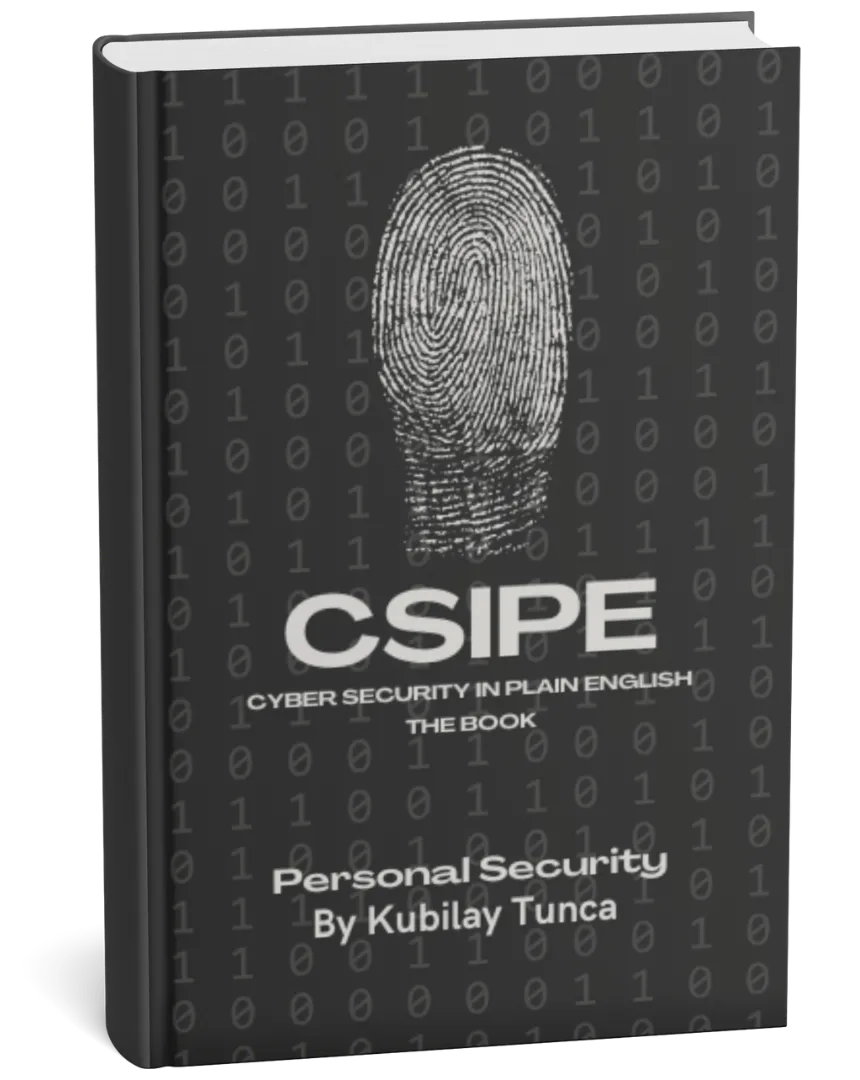
Protect Your Digital Life, Hassle-Free
Private Security in Plain English is your friendly guide to stopping common cyber threats—no tech degree required. Learn the simple, practical steps to safeguard your passwords, devices, and personal data in a language you’ll actually understand.
Buy the Ebook NowIntroduction
Secure defaults, also known as “secure by default,” refer to configurations that prioritize security out of the box. While many popular frameworks include security features, they may not be enabled or configured optimally by default. Developers must proactively implement secure defaults to minimize vulnerabilities and ensure their applications are resilient against attacks.
This guide explores how to configure secure defaults in widely used frameworks, providing actionable steps to bolster application security.
Why Secure Defaults Matter
Secure defaults help mitigate vulnerabilities by establishing a strong baseline for application security. Without them, developers may inadvertently leave critical areas exposed due to misconfigurations or oversight.
Benefits of Secure Defaults:
- Reduced Attack Surface:
- Disabling unused features and enforcing secure practices limits opportunities for exploitation.
- Compliance:
- Many security standards, such as OWASP and PCI DSS, emphasize secure default configurations.
- Enhanced User Trust:
- Secure applications instill confidence in users and stakeholders.
Secure Defaults in Popular Frameworks
1. Django (Python)
Django is known for its strong security features, but developers need to ensure the correct settings are applied.
Key Configurations:
-
CSRF Protection:
-
Enabled by default, but ensure it is not disabled in views or forms.
-
Use the
@csrf_protect
decorator in custom views. -
Secure Cookies:
-
Set the
SESSION_COOKIE_SECURE
andCSRF_COOKIE_SECURE
flags toTrue
to enforce HTTPS.
SESSION_COOKIE_SECURE = True
CSRF_COOKIE_SECURE = True
- Content Security Policy (CSP):
- Use the
django-csp
package to define and enforce CSP rules.
CSP_DEFAULT_SRC = ["'self'"]
CSP_SCRIPT_SRC = ["'self'", "https://trusted-cdn.com"]
2. Express (Node.js)
Express is a lightweight and flexible framework, but developers must configure additional security layers.
Key Configurations:
- HTTP Headers:
- Use the
helmet
middleware to set secure HTTP headers.
const helmet = require('helmet')
app.use(helmet())
- Rate Limiting:
- Prevent abuse by limiting the number of requests per user.
const rateLimit = require('express-rate-limit')
const limiter = rateLimit({
windowMs: 15 * 60 * 1000,
max: 100
})
app.use(limiter)
- Input Validation:
- Use libraries like
express-validator
to sanitize and validate user inputs.
const { check, validationResult } = require('express-validator')
app.post(
'/data',
[check('email').isEmail(), check('age').isInt({ min: 1, max: 120 })],
(req, res) => {
const errors = validationResult(req)
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() })
}
}
)
3. Spring Boot (Java)
Spring Boot provides numerous security features, but developers need to ensure proper configurations.
Key Configurations:
- Enable HTTPS:
- Configure HTTPS using SSL/TLS certificates.
server.ssl.key-store=keystore.p12
server.ssl.key-store-password=yourpassword
server.ssl.keyStoreType=PKCS12
server.port=8443
- CSRF Protection:
- Enabled by default but verify it is not disabled unintentionally.
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().enable();
}
}
- Content Security Policy (CSP):
- Define CSP headers to restrict resource loading.
headers().contentSecurityPolicy("default-src 'self'; script-src 'self' https://trusted-cdn.com");
4. Laravel (PHP)
Laravel includes many built-in security features that need proper configuration.
Key Configurations:
- Input Validation:
- Use Laravel’s validation rules to enforce input constraints.
$request->validate([
'email' => 'required|email',
'password' => 'required|min:8'
]);
- Secure Cookies:
- Set cookies to use HTTPS.
'secure' => env('SESSION_SECURE_COOKIE', true),
- Rate Limiting:
- Use middleware to throttle requests.
Route::middleware('throttle:60,1')->group(function () {
// Routes here
});
5. Angular (JavaScript Framework)
Angular provides client-side security features, but developers must configure them correctly.
Key Configurations:
-
Cross-Site Scripting (XSS) Protection:
-
Angular automatically escapes templates, but avoid bypassing this with functions like
bypassSecurityTrustHtml
. -
Content Security Policy (CSP):
-
Configure CSP headers to restrict resource loading.
-
HTTP Interceptors:
-
Use interceptors to add security headers to API requests.
@Injectable()
export class AuthInterceptor implements HttpInterceptor {
intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
const secureReq = req.clone({
setHeaders: { Authorization: `Bearer ${authToken}` }
})
return next.handle(secureReq)
}
}
Testing and Verifying Secure Defaults
Tools for Testing:
- OWASP ZAP:
- Scan applications for vulnerabilities and misconfigurations.
- Burp Suite:
- Perform penetration testing to identify weak points.
- Linters and Validators:
- Use tools like
eslint
(JavaScript) orflake8
(Python) to enforce coding standards.
Manual Verification:
- Review configuration files for missing or weak settings.
- Test all endpoints for security headers and CSP compliance.
Challenges and Solutions
Challenge: Complexity of Configurations
Solution:
- Use starter templates or baseline configurations provided by frameworks.
Challenge: Keeping Frameworks Updated
Solution:
- Regularly monitor framework updates and apply security patches promptly.
Conclusion
Implementing secure defaults in popular frameworks is a proactive step toward reducing vulnerabilities and building robust applications. By configuring features like HTTPS, CSRF protection, and input validation, developers can significantly enhance their application’s security posture.
Start securing your frameworks today to protect your applications against evolving threats and ensure user trust.