Published
- 4 min read
Writing Secure Shell Scripts for Automation
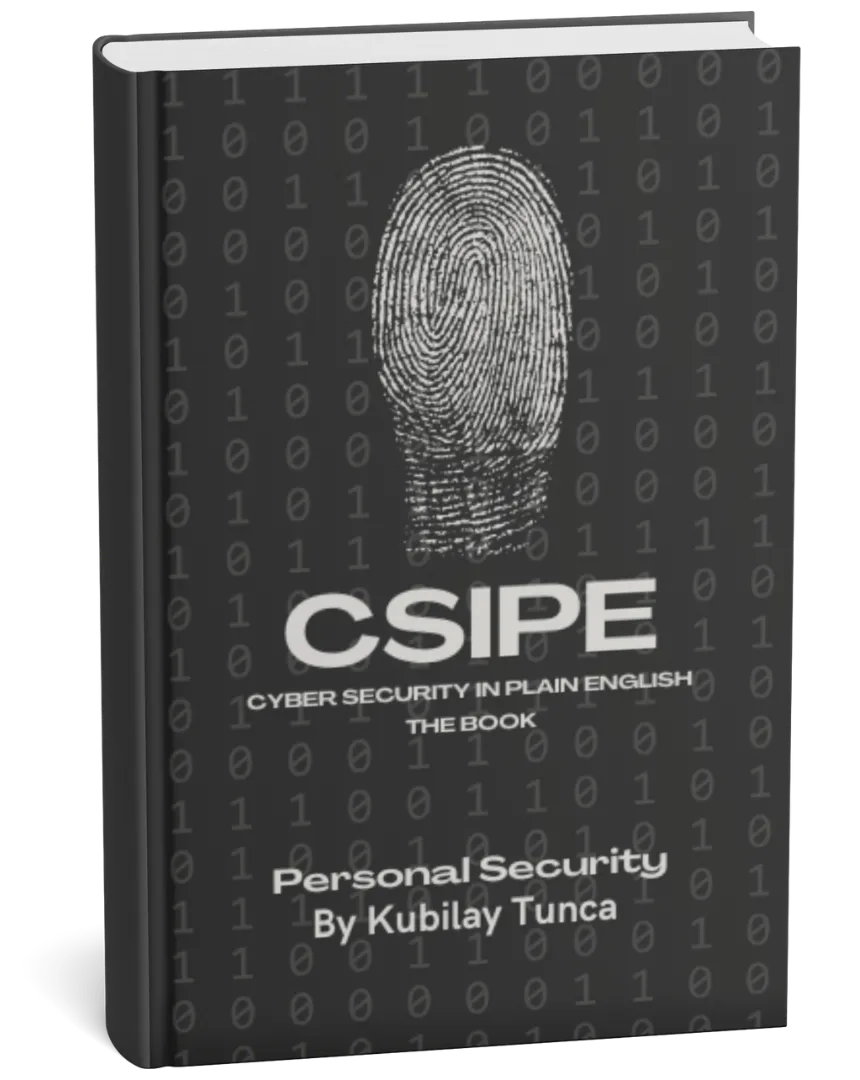
Protect Your Digital Life, Hassle-Free
Private Security in Plain English is your friendly guide to stopping common cyber threats—no tech degree required. Learn the simple, practical steps to safeguard your passwords, devices, and personal data in a language you’ll actually understand.
Buy the Ebook NowIntroduction
Shell scripting is a powerful tool for automating repetitive tasks, managing system configurations, and streamlining workflows. However, insecure shell scripts can inadvertently expose systems to vulnerabilities, such as command injection, privilege escalation, and data leaks. Writing secure shell scripts is essential for developers and system administrators who aim to balance functionality and security.
This comprehensive guide provides best practices, examples, and tools for writing secure shell scripts, ensuring that your automation workflows remain robust and protected.
The Importance of Secure Shell Scripting
1. Prevents Command Injection
Poorly written scripts can allow attackers to inject malicious commands, compromising the system.
2. Protects Sensitive Data
Scripts often handle sensitive information like credentials or configuration files, making secure practices critical.
3. Mitigates Privilege Escalation Risks
Scripts executed with elevated privileges can be exploited if not securely coded.
4. Ensures Compliance
Secure scripting practices help organizations meet regulatory requirements for data security.
Common Security Pitfalls in Shell Scripts
1. Hardcoding Sensitive Data
Embedding passwords, tokens, or API keys directly in scripts increases the risk of exposure.
2. Improper Input Handling
Failing to validate or sanitize user inputs can lead to command injection or unexpected behavior.
3. Overly Broad Permissions
Scripts that require elevated privileges for unnecessary tasks can increase the attack surface.
4. Insecure File Handling
Using predictable file names or insecure directories can allow unauthorized access to temporary or log files.
Best Practices for Writing Secure Shell Scripts
1. Avoid Hardcoding Credentials
What to Do:
- Use environment variables or configuration files with restricted access to store sensitive data.
Example:
#!/bin/bash
# Load credentials from a secure file
source /path/to/secure/config.env
# Use credentials securely
curl -u "$API_USER:$API_PASS" https://api.example.com/data
2. Validate and Sanitize Inputs
What to Do:
- Validate inputs using regex or predefined formats.
- Escape special characters to prevent command injection.
Example:
#!/bin/bash
read -p "Enter a username: " user
# Validate input
if [[ ! "$user" =~ ^[a-zA-Z0-9_]+$ ]]; then
echo "Invalid username."
exit 1
fi
# Use sanitized input
useradd "$user"
3. Use Quotes and Escaping
What to Do:
- Always quote variables to prevent word splitting and globbing.
Example:
#!/bin/bash
filename="/path/to/file name with spaces.txt"
cat "$filename"
4. Limit Privileged Operations
What to Do:
- Use
sudo
for specific commands instead of running the entire script as root.
Example:
#!/bin/bash
# Run privileged operation only when necessary
sudo apt-get update
5. Handle Errors Gracefully
What to Do:
- Use error handling to manage unexpected conditions and avoid exposing sensitive information.
Example:
#!/bin/bash
set -euo pipefail
trap 'echo "An error occurred. Exiting..."; exit 1' ERR
# Commands
cp /important/file /backup/dir
6. Secure Temporary Files
What to Do:
- Use secure directories like
/tmp
ormktemp
for temporary files. - Avoid predictable file names.
Example:
#!/bin/bash
tempfile=$(mktemp)
# Write data to a secure temp file
echo "Temporary data" > "$tempfile"
7. Log Securely
What to Do:
- Mask sensitive information in logs.
- Use restricted access for log files.
Example:
#!/bin/bash
# Redirect logs to a secure location
exec > /var/log/secure_script.log 2>&1
8. Audit and Test Scripts
What to Do:
- Use shellcheck to identify common scripting issues.
- Test scripts in isolated environments before deployment.
Example:
# Install shellcheck
sudo apt-get install shellcheck
# Analyze script
shellcheck my_script.sh
Tools for Secure Shell Scripting
1. ShellCheck
A static analysis tool that detects issues and provides recommendations for shell scripts.
2. Bash Strict Mode
Combines options like set -euo pipefail
to enforce better scripting practices.
3. Ansible (for complex tasks)
Replace lengthy shell scripts with configuration management tools like Ansible for better security and scalability.
Real-World Use Cases
Use Case 1: Automated Database Backup
Problem:
A database backup script was hardcoding credentials, exposing them to potential attackers.
Solution:
- Moved credentials to a secure configuration file with restricted access.
- Used
mktemp
for temporary files.
Result: Reduced the risk of credential exposure and improved script security.
Use Case 2: Secure Deployment Pipeline
Problem:
Deployment scripts were using unvalidated inputs, allowing potential command injection.
Solution:
- Added input validation and error handling.
- Limited privileged operations to specific tasks.
Result: Enhanced security of the deployment process, reducing downtime and vulnerabilities.
Future Trends in Secure Scripting
1. AI-Powered Code Analysis
AI tools will provide real-time suggestions and fixes for scripting vulnerabilities.
2. Integrated Security Frameworks
Security modules will be integrated into shell environments for proactive protection.
3. Zero-Trust Automation
Scripts will adopt zero-trust principles, requiring explicit authentication and access controls for all operations.
Conclusion
Writing secure shell scripts is essential for automating tasks without compromising security. By following the best practices outlined in this guide—such as validating inputs, limiting privileges, and using tools like ShellCheck—you can create robust scripts that protect your systems and data. Start applying these principles today to enhance the security and reliability of your automation workflows.