Published
- 4 min read
Implementing Secure File Uploads in Web Applications
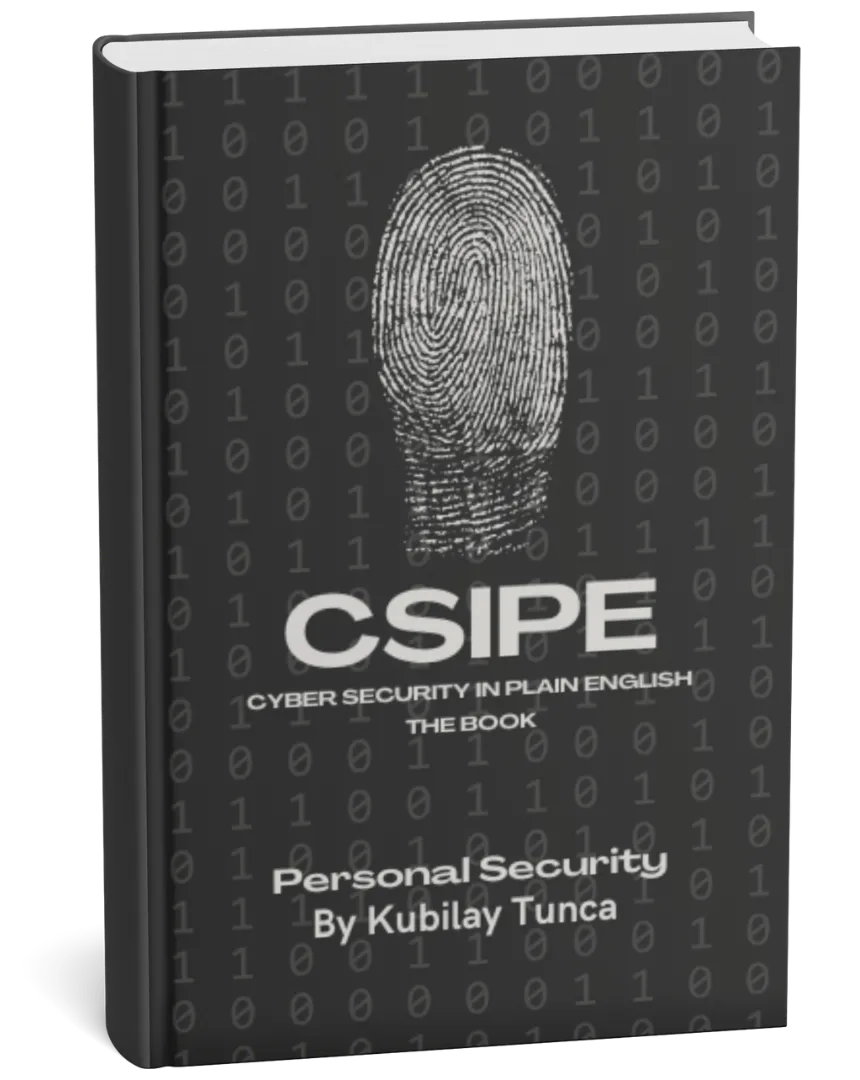
Protect Your Digital Life, Hassle-Free
Private Security in Plain English is your friendly guide to stopping common cyber threats—no tech degree required. Learn the simple, practical steps to safeguard your passwords, devices, and personal data in a language you’ll actually understand.
Buy the Ebook NowIntroduction
File uploads are a common feature in modern web applications, enabling users to upload images, documents, and other content. However, improperly handling file uploads can expose your application to significant security risks, including malware injection, unauthorized file access, and server-side vulnerabilities.
This article explores best practices, tools, and techniques for implementing secure file uploads in web applications. We’ll cover file validation, sanitization, storage strategies, and the use of modern security tools to safeguard your system.
Why Secure File Uploads Matter
1. Preventing Malware Attacks
Attackers can upload malicious files, such as scripts or executables, that exploit server vulnerabilities.
2. Mitigating Unauthorized Access
Poorly configured file upload systems may inadvertently expose sensitive files to unauthorized users.
3. Maintaining Application Integrity
Insecure file uploads can lead to directory traversal attacks, enabling attackers to access restricted areas of your application.
4. Ensuring User Trust
A secure file upload system reassures users that their data is handled safely, enhancing trust and user retention.
Common Security Risks in File Uploads
1. Malicious File Content
Files containing executable code or scripts can compromise your server or client systems.
2. File Overwriting
Improper handling of file names can lead to overwriting existing files, resulting in data loss or security breaches.
3. Directory Traversal
Manipulated file paths can allow attackers to access files outside the intended directory.
4. Excessive File Size
Large files can cause denial-of-service (DoS) attacks by overwhelming server resources.
5. MIME Type Mismatch
Attackers may disguise malicious files as legitimate file types by manipulating MIME headers.
Best Practices for Secure File Uploads
1. Restrict File Types
What to Do:
- Allow only specific file types (e.g.,
.jpg
,.png
,.pdf
) based on your application’s requirements.
Implementation Example:
const allowedFileTypes = ['image/jpeg', 'image/png', 'application/pdf']
app.post('/upload', (req, res) => {
const file = req.files.uploadedFile
if (!allowedFileTypes.includes(file.mimetype)) {
return res.status(400).send('Invalid file type.')
}
// Proceed with file handling
})
2. Validate File Names
What to Do:
- Reject files with special characters or long names to prevent path traversal attacks.
Implementation Example:
const sanitizeFilename = (filename) => filename.replace(/[^a-zA-Z0-9.]/g, '')
app.post('/upload', (req, res) => {
const sanitizedFilename = sanitizeFilename(req.files.uploadedFile.name)
const uploadPath = `/uploads/${sanitizedFilename}`
// Proceed with file handling
})
3. Check File Content
What to Do:
- Inspect file content to ensure it matches the claimed file type.
Implementation Example:
const fileType = require('file-type')
app.post('/upload', async (req, res) => {
const fileBuffer = req.files.uploadedFile.data
const detectedType = await fileType.fromBuffer(fileBuffer)
if (!detectedType || !allowedFileTypes.includes(detectedType.mime)) {
return res.status(400).send('File content does not match type.')
}
// Proceed with file handling
})
4. Limit File Size
What to Do:
- Enforce a maximum file size limit to prevent DoS attacks.
Implementation Example:
const multer = require('multer')
const upload = multer({
limits: { fileSize: 5 * 1024 * 1024 } // 5MB limit
})
app.post('/upload', upload.single('uploadedFile'), (req, res) => {
res.send('File uploaded successfully.')
})
5. Store Files Securely
What to Do:
- Use a dedicated storage solution, such as Amazon S3 or Google Cloud Storage, to keep files separate from application servers.
Implementation Example with AWS S3:
const AWS = require('aws-sdk')
const s3 = new AWS.S3()
app.post('/upload', async (req, res) => {
const params = {
Bucket: 'your-bucket-name',
Key: req.files.uploadedFile.name,
Body: req.files.uploadedFile.data
}
try {
await s3.upload(params).promise()
res.send('File uploaded successfully.')
} catch (error) {
res.status(500).send('Error uploading file.')
}
})
6. Use Temporary Directories
What to Do:
- Store uploaded files in a temporary directory for further validation before moving them to permanent storage.
Example:
const fs = require('fs')
const tempDir = '/tmp/uploads'
if (!fs.existsSync(tempDir)) {
fs.mkdirSync(tempDir)
}
app.post('/upload', (req, res) => {
const tempPath = `${tempDir}/${req.files.uploadedFile.name}`
req.files.uploadedFile.mv(tempPath, (err) => {
if (err) return res.status(500).send('Error saving file.')
// Proceed with further processing
})
})
7. Implement Access Controls
What to Do:
- Restrict access to uploaded files using role-based permissions.
Example:
app.get('/uploads/:filename', (req, res) => {
const user = req.user
if (!user || !user.permissions.includes('view-files')) {
return res.status(403).send('Access denied.')
}
res.sendFile(`/uploads/${req.params.filename}`)
})
Tools for Secure File Uploads
- Multer
- A middleware for handling multipart/form-data in Node.js.
- FileType
- A library for detecting file types from binary content.
- AWS S3
- A secure storage service for handling file uploads.
- ClamAV
- An open-source antivirus engine for scanning uploaded files.
Real-World Use Cases
Use Case 1: Image Uploads for User Profiles
- Problem: Users upload images with malicious scripts.
- Solution: Validate file type, sanitize file names, and store files in a secure S3 bucket.
Use Case 2: Document Submission for Financial Applications
- Problem: Large file uploads cause server crashes.
- Solution: Limit file size, use temporary storage, and enforce content validation.
Future Trends in Secure File Uploads
- AI-Based File Scanning
- AI algorithms will analyze file content for threats in real time.
- Serverless File Handling
- Use serverless architectures to isolate file uploads from application servers.
- Zero-Trust Storage Solutions
- Apply zero-trust principles to file access and storage.
Conclusion
Implementing secure file uploads in web applications is a multi-faceted process that requires robust validation, sanitization, and storage practices. By following the best practices outlined in this guide and leveraging the right tools, you can build a secure file upload system that protects your application and its users. Start securing your file uploads today to prevent vulnerabilities and ensure data integrity.