Published
- 4 min read
How to Implement OAuth2 in Your Application
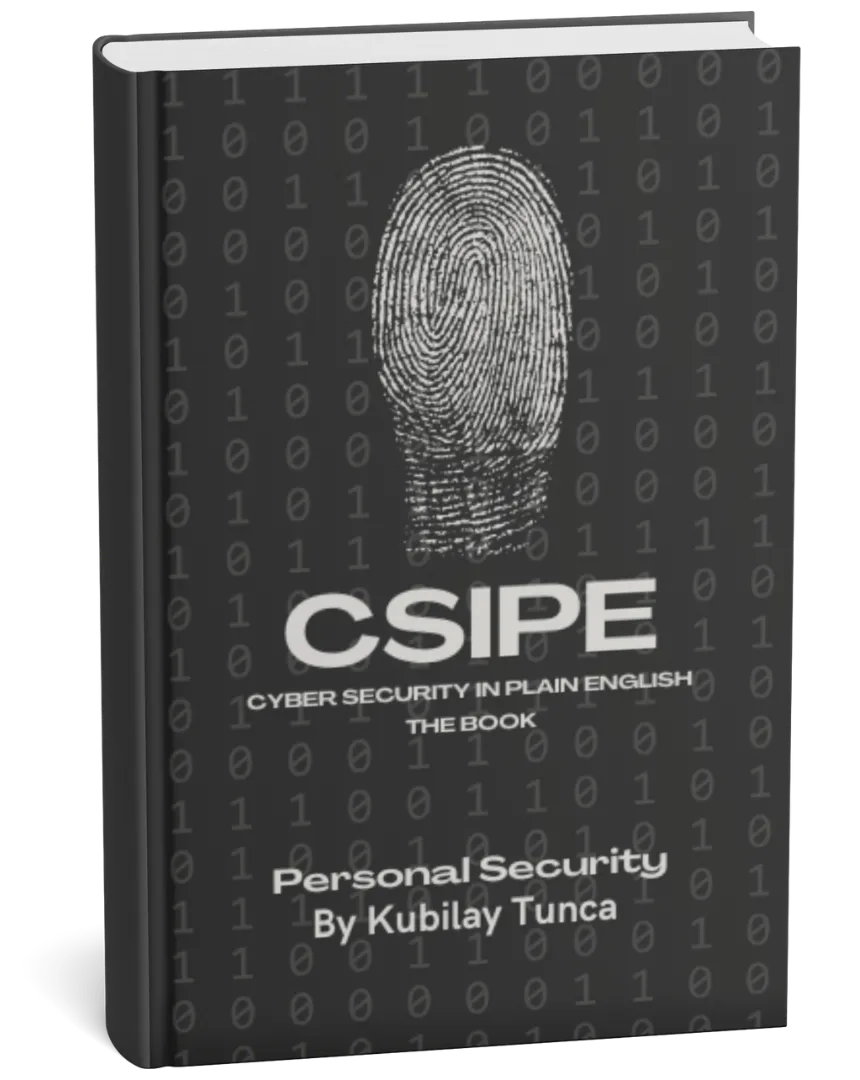
Protect Your Digital Life, Hassle-Free
Private Security in Plain English is your friendly guide to stopping common cyber threats—no tech degree required. Learn the simple, practical steps to safeguard your passwords, devices, and personal data in a language you’ll actually understand.
Buy the Ebook NowIntroduction
OAuth2 is a widely adopted authorization framework that provides secure and scalable mechanisms for enabling third-party access to user resources. Whether you’re building APIs, web applications, or mobile apps, integrating OAuth2 ensures robust authentication and access management while minimizing security risks.
This article serves as a comprehensive guide to implementing OAuth2 in your application, covering its core concepts, architecture, implementation steps, and best practices.
Understanding OAuth2
What Is OAuth2?
OAuth2 is an open standard for delegated authorization, allowing users to grant applications limited access to their resources without exposing their credentials.
Key Components:
- Resource Owner: The user who owns the resources being accessed.
- Client: The application requesting access to the resources.
- Authorization Server: Issues access tokens after authenticating the resource owner.
- Resource Server: Hosts the user’s resources and verifies access tokens.
OAuth2 Authorization Flows
1. Authorization Code Flow
- Use Case: Web applications with a backend server.
- How It Works:
- User logs in via the authorization server.
- Server exchanges an authorization code for an access token.
- Access token is used to access resources.
2. Implicit Flow
- Use Case: Single-page applications (SPA).
- How It Works:
- Access token is issued directly to the client, skipping the authorization code step.
- Note: Considered less secure due to exposure of the token in URLs.
3. Client Credentials Flow
- Use Case: Server-to-server communication (e.g., API integrations).
- How It Works:
- Client authenticates directly with the authorization server using client credentials.
4. Password Grant Flow
- Use Case: Trusted applications (should be avoided if possible).
- How It Works:
- User provides their credentials directly to the client, which exchanges them for an access token.
Implementing OAuth2: A Step-by-Step Guide
Step 1: Register Your Application
- Create an OAuth2 Application
- Register your application with the chosen authorization server (e.g., Google, GitHub, Auth0).
- Obtain client ID and client secret.
- Configure Redirect URIs
- Specify the URLs where users will be redirected after authentication.
Step 2: Implement the Authorization Flow
Example: Authorization Code Flow
- Redirect User to Authorization Server
const clientId = 'your-client-id'
const redirectUri = 'https://yourapp.com/callback'
const authUrl = `https://authserver.com/authorize?response_type=code&client_id=${clientId}&redirect_uri=${redirectUri}`
window.location.href = authUrl
- Exchange Authorization Code for Access Token
import requests
token_url = 'https://authserver.com/token'
client_id = 'your-client-id'
client_secret = 'your-client-secret'
authorization_code = 'received-code'
data = {
'grant_type': 'authorization_code',
'code': authorization_code,
'redirect_uri': 'https://yourapp.com/callback',
'client_id': client_id,
'client_secret': client_secret
}
response = requests.post(token_url, data=data)
access_token = response.json().get('access_token')
- Access Protected Resources
headers = {'Authorization': f'Bearer {access_token}'}
resource_url = 'https://api.resource.com/userinfo'
response = requests.get(resource_url, headers=headers)
print(response.json())
Step 3: Secure Your Implementation
Best Practices:
- Use HTTPS: Encrypt communication to prevent token interception.
- Validate Tokens: Ensure tokens are valid and unexpired using introspection endpoints.
- Use Short-Lived Tokens: Limit the lifespan of access tokens and issue refresh tokens for extended access.
Common Challenges and How to Overcome Them
1. Token Leakage
- Cause: Exposure of tokens in URLs or logs.
- Solution: Store tokens securely (e.g., HTTP-only cookies or secure storage).
2. Misconfigured Scopes
- Cause: Overly broad or undefined access scopes.
- Solution: Restrict access to the minimum necessary scopes.
3. Replay Attacks
- Cause: Reuse of intercepted tokens.
- Solution: Implement nonce values and monitor for suspicious activity.
Tools and Libraries for OAuth2 Integration
1. OAuth2 Libraries
- Node.js: Passport.js (OAuth2 Strategy)
- Python: Authlib, Requests-OAuthlib
- Java: Spring Security OAuth2
2. Authorization Servers
- Auth0: Full-featured identity platform.
- Keycloak: Open-source identity and access management solution.
- AWS Cognito: Managed authentication service.
3. Token Introspection Tools
- jwt.io: Decode and verify JSON Web Tokens (JWTs).
- Postman: Test API endpoints with token-based authentication.
Real-World Use Cases
Use Case 1: Social Media Login
A blogging platform uses Google OAuth2 to enable users to sign in with their Google accounts, streamlining registration and improving user experience.
Use Case 2: API Integration
A SaaS application integrates with a third-party CRM using the client credentials flow, ensuring secure data exchange.
Use Case 3: Multi-Tenant Application
An enterprise platform uses OAuth2 scopes to differentiate access levels for users in various organizations.
Future Trends in OAuth2
1. OAuth2.1
The evolution of OAuth2 with enhanced security and simplified flows, addressing known vulnerabilities in earlier versions.
2. Integration with Zero-Trust Architectures
OAuth2 will play a critical role in enabling granular access controls in zero-trust environments.
3. Enhanced Developer Tooling
More libraries and tools will emerge to simplify OAuth2 implementation and monitoring.
Conclusion
OAuth2 is an essential framework for secure authentication and authorization in modern applications. By understanding its flows, implementing best practices, and leveraging the right tools, developers can build robust, secure, and user-friendly systems. Begin integrating OAuth2 today to ensure your applications meet the highest security standards.