Published
- 4 min read
Building Secure APIs with GraphQL
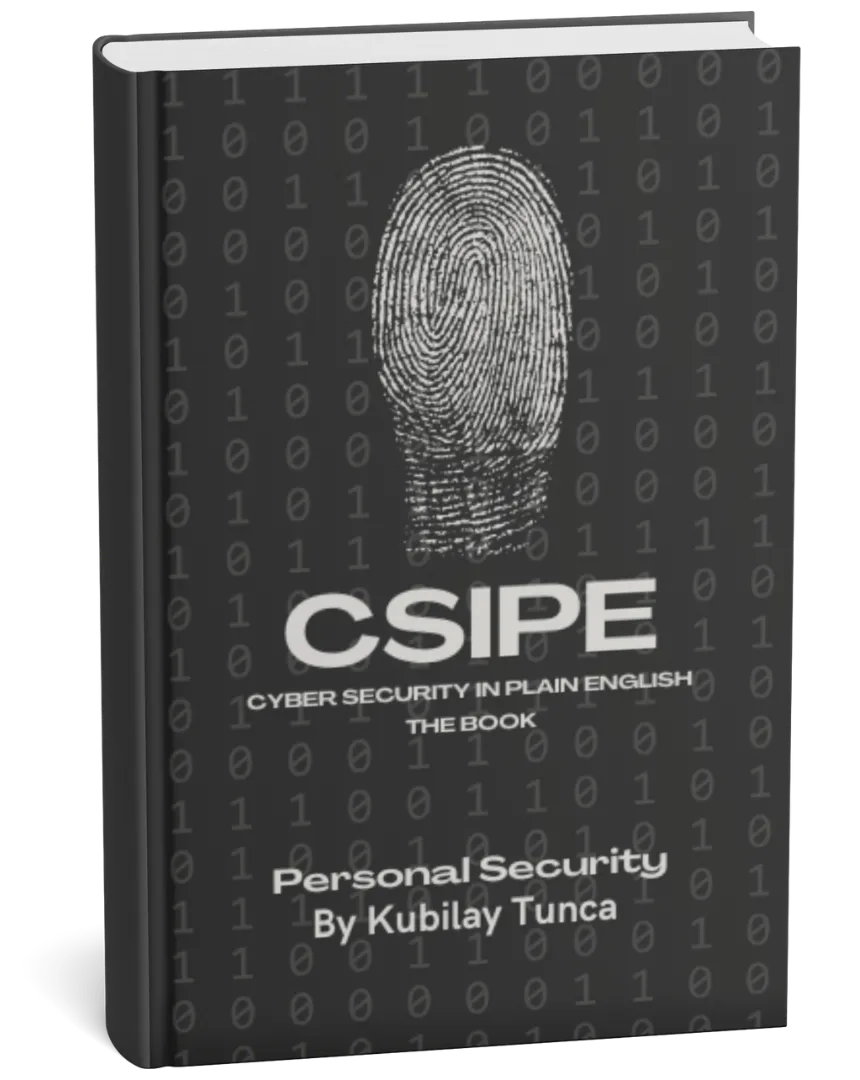
Protect Your Digital Life, Hassle-Free
Private Security in Plain English is your friendly guide to stopping common cyber threats—no tech degree required. Learn the simple, practical steps to safeguard your passwords, devices, and personal data in a language you’ll actually understand.
Buy the Ebook NowIntroduction
GraphQL has revolutionized the way APIs are designed, offering flexibility and efficiency by allowing clients to request exactly the data they need. However, its dynamic nature introduces unique security challenges, such as over-fetching, query complexity, and unauthorized access. This article provides a comprehensive guide to building secure GraphQL APIs, focusing on techniques like validation, authentication, and authorization.
Why Security Is Essential for GraphQL APIs
1. Dynamic Query Execution
GraphQL allows clients to construct their queries dynamically, increasing the risk of abuse through malicious or overly complex queries.
2. Fine-Grained Data Access
While this flexibility is powerful, it requires careful control to prevent unauthorized data exposure.
3. Increased Attack Surface
The schema, resolvers, and underlying systems all need to be secured to protect the API.
Architecture of a Secure GraphQL API
- Frontend Client:
- Sends structured GraphQL queries.
- Handles authentication tokens for API access.
- GraphQL Server:
- Validates incoming queries.
- Enforces authentication and authorization rules.
- Optimizes query resolution to prevent over-fetching.
- Database:
- Ensures secure storage and retrieval of data.
- Middleware:
- Adds layers for rate limiting, query analysis, and logging.
Common GraphQL Security Challenges
1. Over-Querying
- Attackers or clients may request excessive amounts of data, overwhelming the server.
2. Injection Attacks
- Malicious inputs in queries can exploit vulnerabilities in resolvers or underlying databases.
3. Authorization Gaps
- Misconfigured access controls can expose sensitive data to unauthorized users.
4. Lack of Monitoring
- Without proper logging, detecting and mitigating attacks becomes difficult.
Implementing a Secure GraphQL API
Step 1: Schema Design Best Practices
1.1 Avoid Overly Broad Queries
- Design schemas that enforce specificity and limit the amount of data a single query can fetch.
Example:
type User {
id: ID!
email: String!
profile: Profile!
}
type Profile {
bio: String
avatarUrl: String
}
1.2 Leverage Query Depth Limits
- Set depth limits to prevent deeply nested queries.
Implementation (using graphql-depth-limit
):
const depthLimit = require('graphql-depth-limit')
const server = new ApolloServer({
typeDefs,
resolvers,
validationRules: [depthLimit(5)]
})
Step 2: Input Validation
2.1 Validate Query Inputs
- Use tools like Joi or Zod to ensure user inputs meet expected criteria.
Example in Resolver:
const Joi = require('joi')
const schema = Joi.object({
email: Joi.string().email().required(),
password: Joi.string().min(8).required()
})
const resolver = async (parent, args, context) => {
const { error } = schema.validate(args.input)
if (error) throw new Error(error.details[0].message)
// Proceed with logic
}
Step 3: Authentication and Authorization
3.1 Use JSON Web Tokens (JWTs)
- Authenticate users via JWTs in headers and validate them on every request.
Implementation Example:
const jwt = require('jsonwebtoken')
const authenticate = (req) => {
const token = req.headers.authorization
if (!token) throw new Error('Unauthorized')
return jwt.verify(token, process.env.JWT_SECRET)
}
3.2 Implement Role-Based Access Control (RBAC)
- Assign roles to users and restrict access based on roles.
Example in Resolver:
const resolver = async (parent, args, { user }) => {
if (user.role !== 'admin') throw new Error('Access Denied')
// Proceed with logic
}
Step 4: Preventing Over-Querying
4.1 Apply Query Cost Analysis
- Assign a cost to each query field and reject overly expensive queries.
Implementation (using graphql-cost-analysis
):
const costAnalysis = require('graphql-cost-analysis')
const server = new ApolloServer({
typeDefs,
resolvers,
validationRules: [
costAnalysis({
maximumCost: 100,
onComplete: (cost) => console.log('Query cost:', cost)
})
]
})
4.2 Limit Query Complexity
- Combine depth and cost limits for comprehensive protection.
Step 5: Monitoring and Rate Limiting
5.1 Enable Request Logging
- Log incoming queries and their responses for auditing and debugging.
Example with Middleware:
app.use((req, res, next) => {
console.log(`Query: ${req.body.query}`)
next()
})
5.2 Apply Rate Limiting
- Prevent abuse by limiting the number of requests per user.
Implementation (using express-rate-limit
):
const rateLimit = require('express-rate-limit')
const limiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100 // Limit each IP to 100 requests per windowMs
})
app.use(limiter)
Tools for Securing GraphQL APIs
- Apollo Server:
- Provides built-in support for query validation and authentication middleware.
- GraphQL Shield:
- Enables declarative authorization rules.
- DataDog:
- Offers monitoring and alerting for API usage patterns.
- GraphQL Playground:
- Securely test queries with restricted access to sensitive data.
Real-World Use Cases
Use Case 1: E-Commerce Application
An e-commerce platform uses role-based access control (RBAC) to restrict admin-only operations like modifying product inventory.
Use Case 2: Social Media Platform
A social media app applies query cost analysis to prevent users from fetching excessive amounts of data in a single request.
Future Trends in GraphQL Security
- Enhanced Tooling:
- More frameworks will emerge to simplify query cost analysis and depth limiting.
- Federated Security:
- Distributed GraphQL architectures will adopt standardized security practices.
- AI-Powered Monitoring:
- Artificial intelligence will detect and mitigate suspicious query patterns in real time.
Conclusion
Securing a GraphQL API requires a holistic approach, combining schema design, authentication, query analysis, and monitoring. By implementing these best practices and leveraging the right tools, you can protect your API from common vulnerabilities while delivering a seamless experience to your users. Start securing your GraphQL APIs today and build applications that users trust.