Published
- 4 min read
How to Add Multi-Factor Authentication to Your App
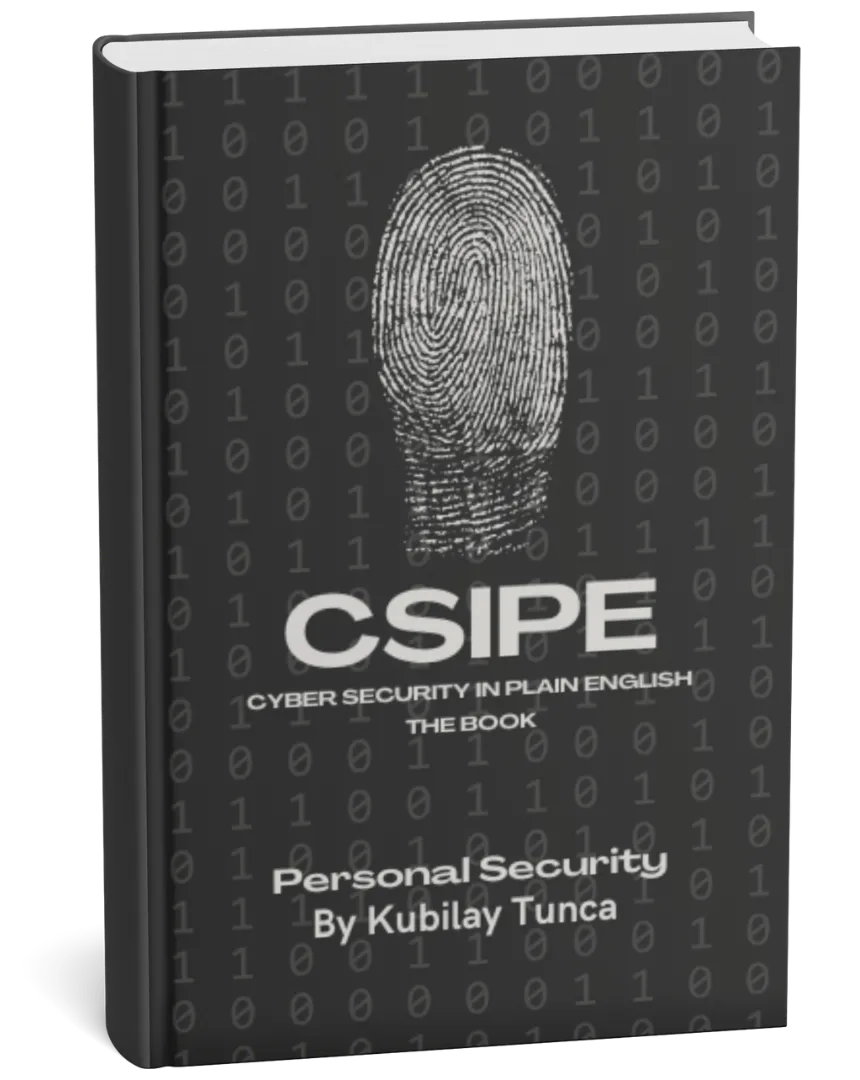
Protect Your Digital Life, Hassle-Free
Private Security in Plain English is your friendly guide to stopping common cyber threats—no tech degree required. Learn the simple, practical steps to safeguard your passwords, devices, and personal data in a language you’ll actually understand.
Buy the Ebook NowIntroduction
With the increasing sophistication of cyberattacks, Multi-Factor Authentication (MFA) has become a cornerstone of application security. By requiring users to provide multiple verification factors, MFA significantly enhances the security of user accounts, protecting them from unauthorized access.
This article provides a comprehensive guide to implementing MFA in your applications, covering its benefits, implementation strategies, and best practices.
Why Multi-Factor Authentication Matters
1. Enhanced Account Security
Passwords alone are no longer sufficient. MFA adds an extra layer of security by requiring a combination of factors, such as something the user knows (password), has (authenticator app or hardware token), or is (biometric verification).
2. Mitigates Common Attacks
MFA protects against password-related attacks, such as brute force, phishing, and credential stuffing.
3. Regulatory Compliance
Many industries require MFA for compliance with standards like GDPR, HIPAA, and PCI DSS.
4. Boosts User Trust
Implementing MFA demonstrates a commitment to user security, fostering trust and confidence.
Types of Multi-Factor Authentication
1. Time-Based One-Time Passwords (TOTP)
- Users receive a time-sensitive code generated by an authenticator app like Google Authenticator or Authy.
- Pros: Easy to implement, widely supported.
- Cons: Requires users to install an app.
2. Push Notifications
- Users approve or deny a login attempt via a mobile notification.
- Pros: Seamless user experience.
- Cons: Requires a reliable mobile app infrastructure.
3. SMS-Based OTP
- Codes are sent via SMS for verification.
- Pros: Universally accessible.
- Cons: Vulnerable to SIM-swapping attacks.
4. Hardware Tokens
- Users carry physical devices, such as YubiKeys, for authentication.
- Pros: Highly secure.
- Cons: Additional cost for hardware.
5. Biometric Authentication
- Relies on physical traits like fingerprints, facial recognition, or voice patterns.
- Pros: Convenient and secure.
- Cons: Requires specialized hardware.
Implementation Steps for MFA
Step 1: Set Up the Backend
1.1 Install Dependencies
npm init -y
npm install express bcrypt jsonwebtoken speakeasy qrcode
1.2 Generate TOTP Secrets
const speakeasy = require('speakeasy')
const generateSecret = () => {
const secret = speakeasy.generateSecret({ length: 20 })
console.log('Secret:', secret.base32) // Store this securely
return secret
}
1.3 Verify TOTP Tokens
const verifyToken = (token, secret) => {
const isValid = speakeasy.totp.verify({
secret: secret,
encoding: 'base32',
token: token
})
return isValid
}
Step 2: Add MFA During User Registration
- Generate a TOTP secret for the user.
- Display a QR code for the user to scan with an authenticator app.
Example Code:
const qrcode = require('qrcode')
app.post('/register-mfa', async (req, res) => {
const { email, password } = req.body
const hashedPassword = await bcrypt.hash(password, 10)
const secret = speakeasy.generateSecret()
const qrCodeUrl = await qrcode.toDataURL(secret.otpauth_url)
// Save user and secret to database
res.json({ qrCodeUrl, secret: secret.base32 })
})
Step 3: Verify MFA During Login
- Authenticate the user’s credentials.
- Prompt for an MFA token after successful authentication.
- Verify the token before granting access.
Example Code:
app.post('/login-mfa', async (req, res) => {
const { email, password, token } = req.body
const user = await User.findOne({ email })
if (!user || !(await bcrypt.compare(password, user.password))) {
return res.status(401).send('Invalid credentials')
}
const isTokenValid = speakeasy.totp.verify({
secret: user.mfaSecret,
encoding: 'base32',
token: token
})
if (!isTokenValid) {
return res.status(401).send('Invalid MFA token')
}
const jwtToken = jwt.sign({ id: user._id }, process.env.JWT_SECRET, { expiresIn: '1h' })
res.json({ jwtToken })
})
Step 4: Frontend Integration
4.1 Display the QR Code
Use a library like react-qr-code
to show the QR code during registration.
import QRCode from 'react-qr-code'
function QrCodeDisplay({ url }) {
return <QRCode value={url} />
}
4.2 MFA Login Form
Prompt users for their MFA token after entering their credentials.
import React, { useState } from 'react'
import axios from 'axios'
function MfaLogin() {
const [email, setEmail] = useState('')
const [password, setPassword] = useState('')
const [token, setToken] = useState('')
const handleSubmit = async (e) => {
e.preventDefault()
try {
const response = await axios.post('http://localhost:5000/login-mfa', {
email,
password,
token
})
localStorage.setItem('token', response.data.jwtToken)
alert('Login successful')
} catch (error) {
alert('Authentication failed')
}
}
return (
<form onSubmit={handleSubmit}>
<input
type='email'
placeholder='Email'
value={email}
onChange={(e) => setEmail(e.target.value)}
/>
<input
type='password'
placeholder='Password'
value={password}
onChange={(e) => setPassword(e.target.value)}
/>
<input
type='text'
placeholder='MFA Token'
value={token}
onChange={(e) => setToken(e.target.value)}
/>
<button type='submit'>Login</button>
</form>
)
}
export default MfaLogin
Best Practices for MFA
- Backup Codes
- Provide users with backup codes during registration for recovery purposes.
- Rate Limiting
- Limit failed attempts to prevent brute force attacks on MFA tokens.
- Periodic Reauthentication
- Require users to reauthenticate periodically, especially for sensitive actions.
- Educate Users
- Inform users about the importance of MFA and how to secure their accounts.
Real-World Use Cases
Use Case 1: Securing a Financial Application
A banking app implements TOTP-based MFA to protect user accounts from unauthorized transactions.
Use Case 2: Enterprise Applications
An HR management system integrates hardware tokens for secure employee access.
Future Trends in MFA
- Biometric MFA
- Combining biometrics with traditional MFA factors for enhanced security.
- FIDO2 Authentication
- Passwordless authentication using WebAuthn and hardware keys.
- Adaptive MFA
- Dynamically adjusting MFA requirements based on user behavior and context.
Conclusion
Adding Multi-Factor Authentication to your app is a crucial step in enhancing security and protecting users from unauthorized access. By following the implementation strategies and best practices outlined in this guide, you can create a secure, user-friendly MFA system. Start integrating MFA today to build trust and ensure the safety of your applications.