Published
- 4 min read
Setting Up HTTPS for Local Development
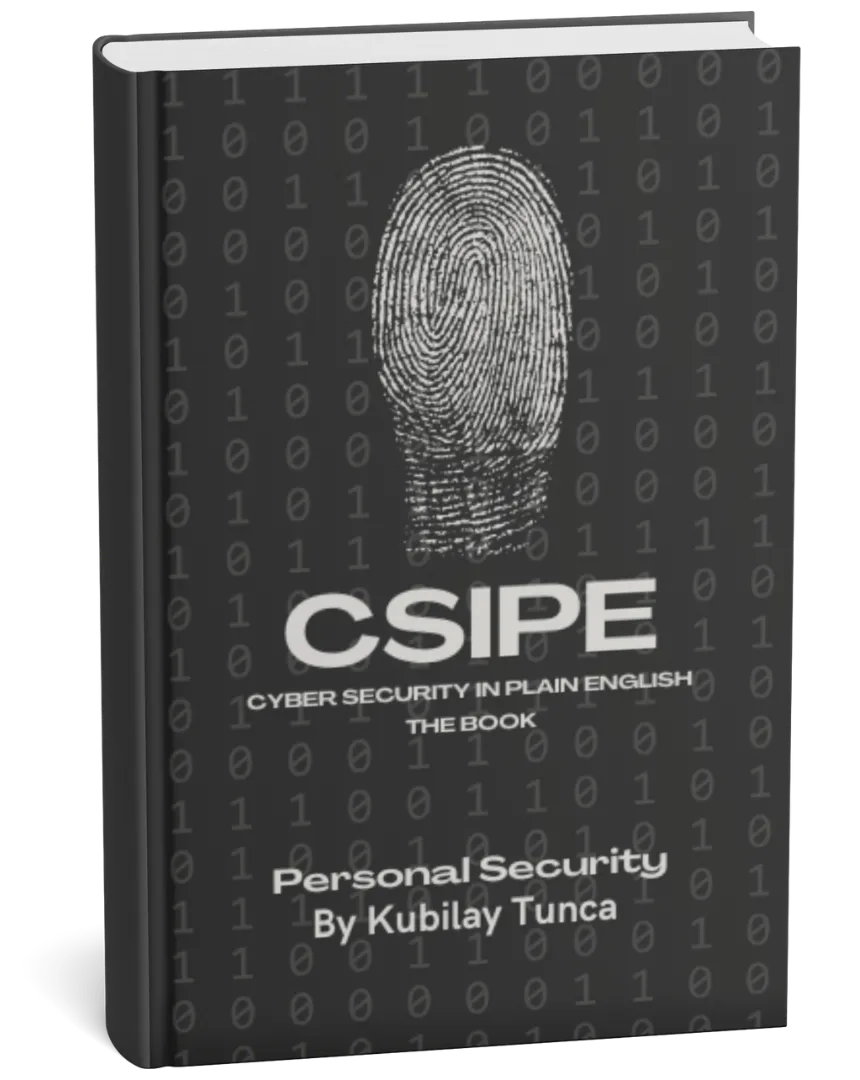
Protect Your Digital Life, Hassle-Free
Private Security in Plain English is your friendly guide to stopping common cyber threats—no tech degree required. Learn the simple, practical steps to safeguard your passwords, devices, and personal data in a language you’ll actually understand.
Buy the Ebook NowIntroduction
In modern web development, HTTPS is essential for securing data in transit, protecting user privacy, and ensuring trust. While HTTPS is standard in production environments, setting it up for local development often gets overlooked. By configuring HTTPS locally, developers can simulate secure environments, test SSL-specific features, and catch potential issues early.
This guide provides a step-by-step approach to setting up HTTPS for local development using self-signed certificates, tools, and best practices.
Why Use HTTPS in Local Development?
1. Simulating Real-World Scenarios
- Testing applications in a secure environment ensures that they work seamlessly in production.
2. Catching SSL/TLS Issues Early
- Identifying HTTPS-related problems during development saves time and reduces deployment risks.
3. Enabling Secure APIs
- Many APIs require HTTPS, even in development, to accept requests.
4. Compliance Testing
- Some features, like browser-based geolocation, require HTTPS to function.
Prerequisites
Tools and Libraries Needed:
- OpenSSL: For generating self-signed certificates.
- Node.js or Python: To run local servers with HTTPS.
- A Web Browser: For testing HTTPS configurations.
Generating a Self-Signed Certificate
Step 1: Install OpenSSL
Install OpenSSL if it’s not already available on your system.
- For macOS: OpenSSL is included. If not, use Homebrew:
brew install openssl
. - For Windows: Download the installer from openssl.org.
- For Linux: Use your package manager, e.g.,
sudo apt-get install openssl
.
Step 2: Create a Certificate and Private Key
Run the following OpenSSL commands to generate a certificate and private key:
openssl req -x509 -nodes -days 365 -newkey rsa:2048 -keyout local-dev.key -out local-dev.crt
Explanation:
-x509
: Creates a self-signed certificate.-nodes
: Prevents encrypting the private key.-days 365
: Specifies the certificate’s validity period.-newkey rsa:2048
: Generates a new RSA key with 2048 bits.
When prompted, provide the following details (use dummy values for local development):
- Country Name:
US
- State:
California
- Locality:
San Francisco
- Organization:
LocalDev
- Common Name:
localhost
This generates two files:
local-dev.key
: The private key.local-dev.crt
: The self-signed certificate.
Step 3: Configure the Development Server
Using Node.js
- Install dependencies:
npm install express https fs
- Create an HTTPS server:
const https = require('https')
const fs = require('fs')
const express = require('express')
const app = express()
const options = {
key: fs.readFileSync('local-dev.key'),
cert: fs.readFileSync('local-dev.crt')
}
app.get('/', (req, res) => {
res.send('Hello, HTTPS World!')
})
https.createServer(options, app).listen(3000, () => {
console.log('HTTPS Server running on https://localhost:3000')
})
- Run the server:
node server.js
Visit https://localhost:3000
in your browser. You’ll see a security warning because the certificate isn’t signed by a trusted authority.
Using Python
- Create a self-signed certificate with OpenSSL.
- Start a Python HTTPS server:
python -m http.server --bind localhost --certfile local-dev.crt --keyfile local-dev.key 8443
Visit https://localhost:8443
in your browser.
Handling Self-Signed Certificate Warnings
Option 1: Add the Certificate to Trusted Authorities
- Add the
local-dev.crt
to your operating system’s trusted root certificates. - macOS: Use Keychain Access.
- Windows: Use the Certificates MMC snap-in.
- Linux: Add it to
/etc/ssl/certs/
.
Option 2: Bypass Warnings (Development Only)
- Manually accept the certificate warning in your browser.
Best Practices for HTTPS in Local Development
- Automate Certificate Generation
- Use tools like
mkcert
to simplify the process of creating local certificates.
- Enable HSTS
- Simulate production behavior by enabling HTTP Strict Transport Security (HSTS).
- Redirect HTTP to HTTPS
- Use middleware to redirect HTTP requests to HTTPS.
Example (Node.js):
app.use((req, res, next) => {
if (!req.secure) {
return res.redirect(`https://${req.headers.host}${req.url}`)
}
next()
})
- Use Secure Cookies
- Mark cookies as
Secure
to ensure they are only sent over HTTPS.
- Monitor for SSL/TLS Issues
- Use browser developer tools to identify insecure resources and mixed content.
Troubleshooting Common Issues
1. Browser Shows “Connection Not Secure”
- Ensure the self-signed certificate is added to trusted authorities.
2. Server Fails to Start
- Verify the paths to
local-dev.key
andlocal-dev.crt
. - Check if the port (e.g.,
3000
or8443
) is already in use.
3. Mixed Content Warnings
- Ensure all assets (e.g., CSS, JS, images) are served over HTTPS.
Real-World Use Cases for HTTPS in Local Development
- Testing Secure APIs
- Simulate production-like HTTPS endpoints for API testing.
- Building Progressive Web Apps (PWAs)
- Many PWA features, like service workers, require HTTPS.
- Developing Secure Authentication Systems
- Ensure features like OAuth2 and JWT-based login work seamlessly in secure environments.
Future Trends in HTTPS for Development
- Automatic Certificate Management
- Tools like
mkcert
and Docker Compose will streamline HTTPS setup for local environments.
- Enhanced Local SSL Tools
- Expect improvements in browser and OS support for local HTTPS testing.
- Integration with Zero-Trust Architectures
- HTTPS will play a crucial role in securing microservices and distributed systems.
Conclusion
Setting up HTTPS for local development is a vital step toward building secure, production-ready applications. By following the steps in this guide, you can simulate secure environments, test SSL-specific features, and avoid potential issues in deployment. Start implementing HTTPS in your local development workflow today and ensure your applications are built with security in mind.