Published
- 3 min read
How to Secure WebSockets in Real-Time Applications
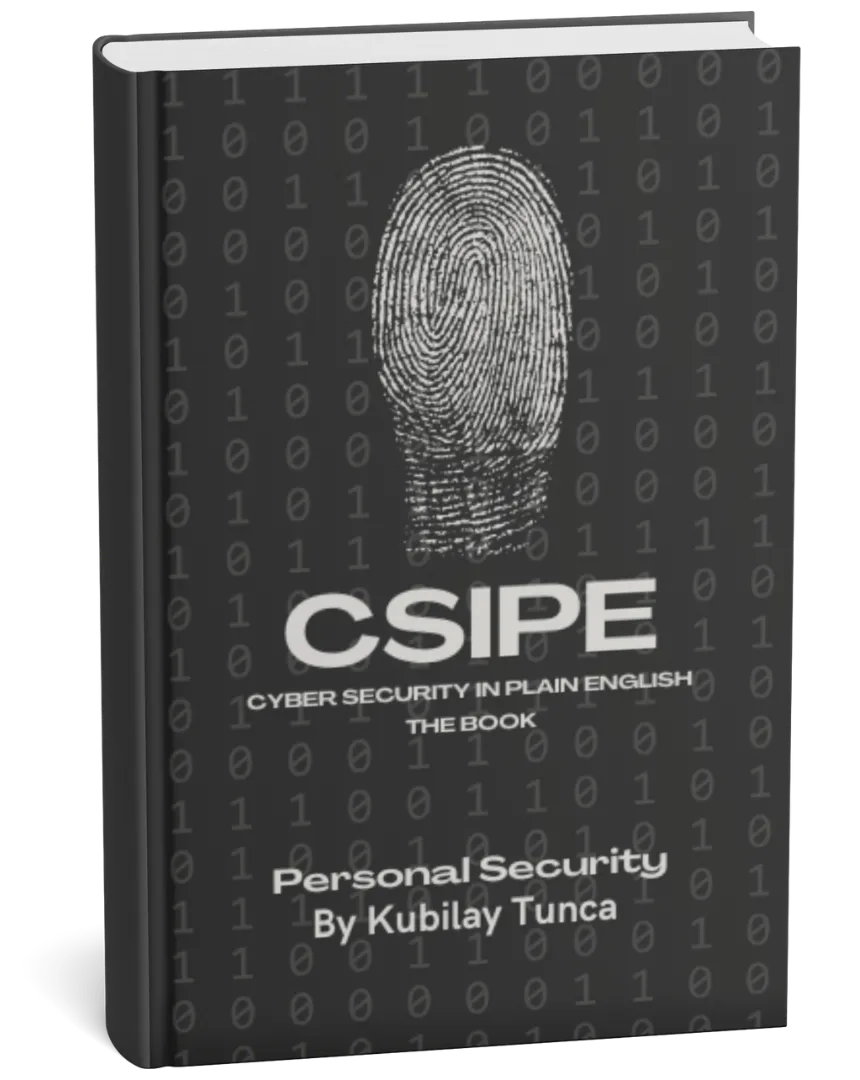
Protect Your Digital Life, Hassle-Free
Private Security in Plain English is your friendly guide to stopping common cyber threats—no tech degree required. Learn the simple, practical steps to safeguard your passwords, devices, and personal data in a language you’ll actually understand.
Buy the Ebook NowIntroduction
WebSockets have revolutionized real-time communication in web applications, providing a persistent connection between clients and servers for seamless data exchange. From chat applications to collaborative tools and live updates, WebSockets empower developers to create responsive and interactive experiences. However, their open and continuous nature makes them susceptible to unique security risks.
This guide explores potential vulnerabilities in WebSocket-based applications and offers practical strategies to secure these real-time connections, ensuring robust protection against exploitation.
Understanding WebSocket Security Risks
WebSockets differ from traditional HTTP protocols by maintaining a long-lived connection, which introduces specific vulnerabilities. Understanding these risks is crucial for developers building secure applications.
Key Security Risks:
- Cross-Site WebSocket Hijacking:
- Unauthorized use of authenticated WebSocket connections by malicious sites.
- Injection Attacks:
- Exploiting unvalidated data sent through WebSocket messages.
- Man-in-the-Middle (MITM) Attacks:
- Interception of unencrypted WebSocket communications.
- DoS Attacks:
- Overloading servers with excessive WebSocket connections.
Strategies for Securing WebSockets
1. Use Secure WebSocket Protocol (WSS)
Always use wss://
for encrypted WebSocket communication over TLS. This protects against eavesdropping and ensures data integrity.
Example (Node.js WebSocket Server with HTTPS):
const https = require('https')
const WebSocket = require('ws')
const fs = require('fs')
const server = https.createServer({
cert: fs.readFileSync('server.cert'),
key: fs.readFileSync('server.key')
})
const wss = new WebSocket.Server({ server })
wss.on('connection', (ws) => {
ws.on('message', (message) => {
console.log('Received:', message)
})
ws.send('Connection secured')
})
server.listen(443, () => {
console.log('Secure WebSocket server running')
})
2. Validate and Sanitize Messages
WebSocket messages often carry dynamic data that must be validated and sanitized to prevent injection attacks.
Example (Message Validation):
wss.on('connection', (ws) => {
ws.on('message', (message) => {
try {
const data = JSON.parse(message)
if (typeof data.action !== 'string' || !data.action.match(/^[a-z]+$/)) {
throw new Error('Invalid data format')
}
// Process valid data
} catch (error) {
ws.send(JSON.stringify({ error: 'Invalid message' }))
}
})
})
3. Implement Authentication and Authorization
Authenticate WebSocket connections using tokens, such as JSON Web Tokens (JWT). Additionally, enforce role-based access control (RBAC) for specific actions.
Example (JWT Authentication):
const jwt = require('jsonwebtoken')
wss.on('connection', (ws, req) => {
const token = req.headers['sec-websocket-protocol']
try {
const user = jwt.verify(token, 'secretKey')
ws.user = user
} catch (error) {
ws.close(1008, 'Unauthorized')
}
})
4. Limit Connections and Message Size
Set thresholds for the number of concurrent connections and the size of messages to mitigate DoS attacks.
Example (Connection Limit):
const MAX_CONNECTIONS = 100
let connectionCount = 0
wss.on('connection', (ws) => {
if (connectionCount >= MAX_CONNECTIONS) {
ws.close(1013, 'Service Unavailable')
return
}
connectionCount++
ws.on('close', () => {
connectionCount--
})
})
5. Monitor and Log Activity
Track WebSocket connections and interactions to detect anomalies or potential attacks. Implement logging for debugging and security audits.
Example (Logging Connections):
wss.on('connection', (ws) => {
console.log(`New connection: ${ws._socket.remoteAddress}`)
ws.on('message', (message) => {
console.log(`Message from ${ws._socket.remoteAddress}: ${message}`)
})
})
Advanced Security Enhancements
Implement Rate Limiting
Prevent abuse by limiting the rate of messages per connection.
Enable Subprotocols
Define subprotocols for WebSocket communication to enforce expected behaviors and structures.
Leverage Secure Origins
Only allow WebSocket connections from trusted origins.
Regularly Patch Dependencies
Keep WebSocket libraries and server software updated to address known vulnerabilities.
Challenges and Solutions
Challenge: Balancing Security with Performance
Solution: Use efficient encryption algorithms and optimize server handling to maintain low latency while ensuring secure communication.
Challenge: Mitigating MITM Risks in Public Networks
Solution: Enforce strict TLS configurations and validate client certificates for sensitive applications.
Challenge: Managing Scalability with Security
Solution: Use load balancers with WebSocket support to distribute connections while maintaining consistent security policies.
Conclusion
Securing WebSockets is essential for building reliable real-time applications in an increasingly interconnected world. By implementing the strategies outlined in this guide—ranging from secure protocols to robust authentication and real-time monitoring—developers can create WebSocket-based applications that are both efficient and resilient against emerging threats.
Start applying these practices today to ensure your real-time applications remain secure and trustworthy, fostering confidence among users and stakeholders alike.