Published
- 4 min read
How to Protect APIs Against DDoS Attacks
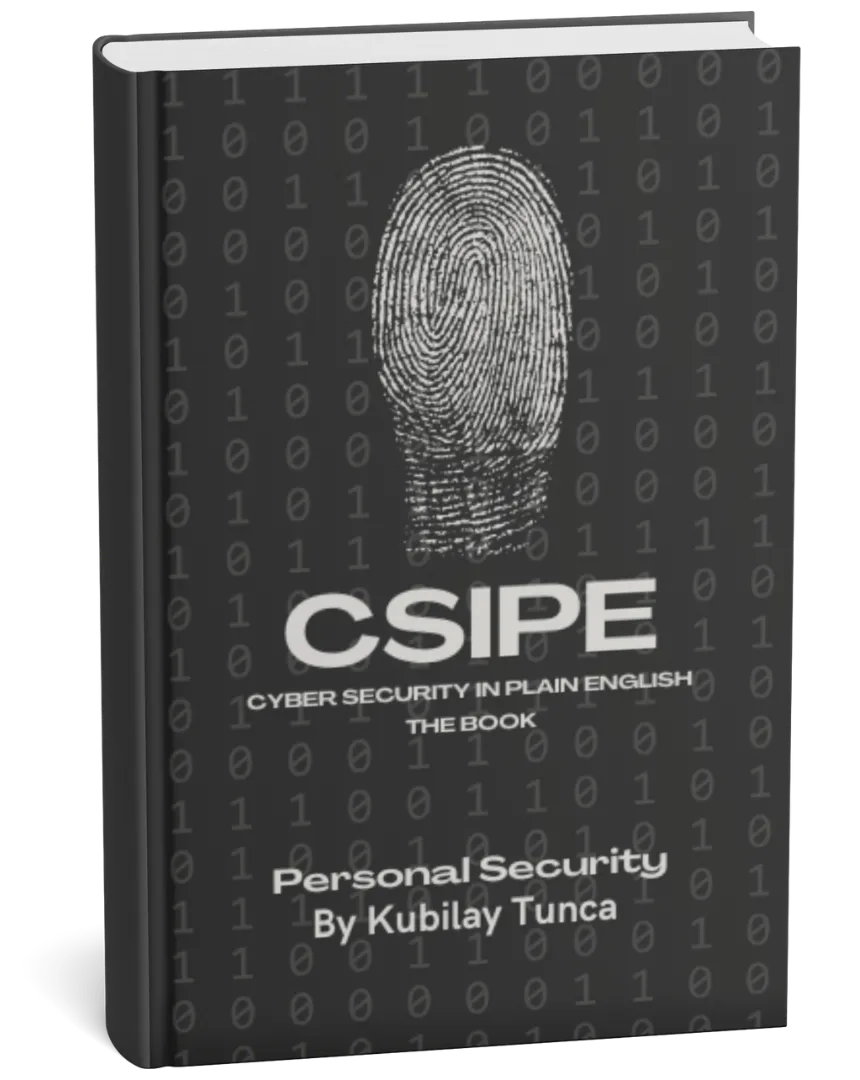
Protect Your Digital Life, Hassle-Free
Private Security in Plain English is your friendly guide to stopping common cyber threats—no tech degree required. Learn the simple, practical steps to safeguard your passwords, devices, and personal data in a language you’ll actually understand.
Buy the Ebook NowIntroduction
In a world driven by interconnected systems and real-time data exchange, APIs play a crucial role in powering web and mobile applications. However, their open and accessible nature makes APIs a common target for Distributed Denial of Service (DDoS) attacks. A successful DDoS attack can overload your API servers, disrupt services, and cause significant downtime.
This guide explores the nuances of DDoS attacks, their impact on APIs, and effective strategies to protect your systems against these threats.
What Are DDoS Attacks?
DDoS attacks overwhelm a server, service, or network by flooding it with an excessive amount of traffic. Attackers typically leverage botnets—a network of compromised devices—to launch simultaneous requests, making it difficult for the target system to differentiate legitimate traffic from malicious requests.
Types of DDoS Attacks on APIs
- Volume-Based Attacks:
- Floods the API server with high volumes of traffic to exhaust bandwidth.
- Protocol Attacks:
- Exploits weaknesses in networking protocols, such as SYN floods or fragmented packet attacks.
- Application Layer Attacks:
- Specifically targets API endpoints, sending legitimate-looking requests designed to exhaust application resources.
The Impact of DDoS Attacks on APIs
The consequences of a DDoS attack extend beyond downtime. They can:
- Degrade User Experience: Slow or unresponsive APIs can frustrate users.
- Financial Losses: Service disruptions lead to lost revenue and potential SLA penalties.
- Brand Damage: Frequent outages can erode customer trust.
- Operational Strain: Recovery efforts consume valuable time and resources.
Strategies to Protect APIs Against DDoS Attacks
1. Rate Limiting
Implement rate limiting to control the number of requests a client can make within a specified time frame. This prevents attackers from overwhelming your API with excessive traffic.
Example (Express.js Middleware):
const rateLimit = require('express-rate-limit')
const limiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100, // Limit each IP to 100 requests per windowMs
message: 'Too many requests from this IP, please try again later.'
})
app.use('/api/', limiter)
2. API Gateway Protection
API gateways act as a buffer between clients and your backend services, providing advanced security features like rate limiting, authentication, and DDoS protection.
Popular API Gateways:
- Amazon API Gateway: Built-in DDoS protection via AWS Shield.
- Kong: Open-source gateway with plugin support.
- Apigee: Offers integrated security and traffic management.
3. Web Application Firewalls (WAFs)
Deploy a WAF to filter and block malicious traffic before it reaches your API endpoints. WAFs analyze traffic patterns, identify anomalies, and enforce security rules.
Example (AWS WAF):
aws wafv2 create-web-acl --name "API-WAF" --scope "REGIONAL" --default-action Allow --rules file://waf-rules.json
4. Distributed Denial of Service (DDoS) Protection Services
Use dedicated DDoS protection services to detect and mitigate attacks in real time. These services, such as AWS Shield, Cloudflare, or Akamai, automatically analyze incoming traffic and block malicious requests.
5. IP Whitelisting
Restrict access to your API by allowing requests only from trusted IP addresses. This strategy is particularly useful for internal or partner-facing APIs.
Example (Nginx Configuration):
location /api/ {
allow 192.168.1.1;
deny all;
}
6. Throttling
Implement throttling mechanisms to slow down excessive traffic. Unlike rate limiting, throttling reduces the speed of responses for high-frequency requests instead of blocking them outright.
7. Token-Based Authentication
Use tokens, such as JSON Web Tokens (JWT), to authenticate API requests. By requiring valid tokens, you can ensure only authorized clients access your API.
Example (JWT Verification in Node.js):
const jwt = require('jsonwebtoken')
app.use('/api/', (req, res, next) => {
const token = req.headers.authorization.split(' ')[1]
jwt.verify(token, 'secretKey', (err, decoded) => {
if (err) {
return res.status(401).send('Unauthorized')
}
req.user = decoded
next()
})
})
8. Logging and Monitoring
Monitor API traffic to detect unusual patterns that could indicate an ongoing DDoS attack. Use tools like Prometheus and Grafana to visualize traffic and set up alerts for anomalies.
Tools for DDoS Protection
Cloud-Based Solutions
- AWS Shield: Provides DDoS protection integrated with other AWS services.
- Cloudflare: Offers robust DDoS mitigation and traffic filtering capabilities.
- Akamai Prolexic: Protects against large-scale DDoS attacks.
Monitoring Tools
- Zeek: Monitors network traffic for anomalies.
- Wireshark: Analyzes packet-level details.
Challenges in Mitigating DDoS Attacks
Balancing Security with Performance
Excessive restrictions can block legitimate users, impacting user experience. To avoid this, implement adaptive rate limiting that accounts for traffic variations.
Evolving Attack Techniques
Attackers continually adapt their strategies to bypass protections. Regularly update your tools and policies to stay ahead of emerging threats.
Cost Management
DDoS protection services can be expensive. Use scalable solutions that grow with your application’s needs.
Conclusion
Protecting APIs against DDoS attacks is essential for maintaining availability, ensuring user satisfaction, and safeguarding your reputation. By implementing a combination of rate limiting, WAFs, API gateways, and monitoring tools, developers can create resilient APIs capable of withstanding even sophisticated attacks.
Start applying these strategies today to fortify your APIs against DDoS threats and ensure uninterrupted service for your users.