Published
- 5 min read
How Encryption Works and Why It’s Essential in Coding
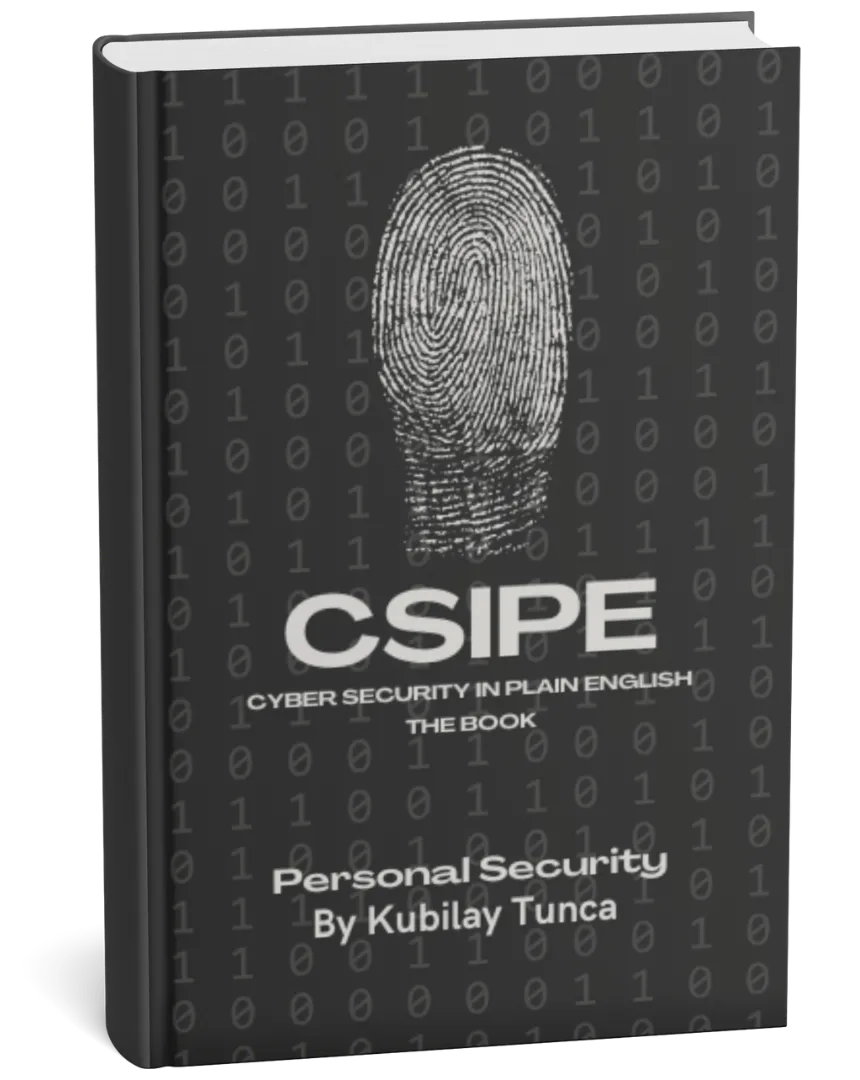
Protect Your Digital Life, Hassle-Free
Private Security in Plain English is your friendly guide to stopping common cyber threats—no tech degree required. Learn the simple, practical steps to safeguard your passwords, devices, and personal data in a language you’ll actually understand.
Buy the Ebook NowIntroduction
Encryption is a cornerstone of modern cybersecurity, serving as a powerful tool to safeguard data from unauthorized access. Whether you’re transmitting sensitive information over the internet or storing user credentials in a database, encryption ensures that your data remains secure. For developers, understanding encryption isn’t just an optional skill—it’s an essential component of building trustworthy, resilient applications.
This article provides an in-depth exploration of encryption, its significance, and how developers can integrate it into their coding practices. From understanding the fundamental principles of encryption to implementing advanced techniques, you’ll gain actionable knowledge to enhance the security of your projects.
What is Encryption?
Encryption is the process of converting plaintext data into an unreadable format (ciphertext) to prevent unauthorized access. Only those with the appropriate decryption key can reverse the process to access the original data. This mechanism is vital for maintaining confidentiality, ensuring data integrity, and building secure systems.
A Brief History of Encryption
Encryption has been around for centuries. Early examples include:
- Caesar Cipher: One of the simplest encryption techniques, used by Julius Caesar to secure messages by shifting letters in the alphabet by a fixed number.
- Enigma Machine: A complex encryption device used by the German military during World War II.
- Data Encryption Standard (DES): Developed in the 1970s, DES became one of the first widely adopted digital encryption algorithms.
Today, modern encryption algorithms are far more sophisticated, relying on mathematical principles and computational power to secure data effectively.
Why Encryption is Essential in Coding
As developers, you handle sensitive data daily—whether it’s user passwords, payment details, or confidential business information. Without encryption, this data is vulnerable to interception, theft, or tampering.
Key Benefits of Encryption in Applications
-
Data Confidentiality: Encryption ensures that sensitive data is accessible only to authorized individuals. For instance, encrypting user credentials protects them from being exposed in the event of a database breach.
-
Data Integrity: Encryption helps verify that data hasn’t been altered during transmission. Any unauthorized modification would render the ciphertext invalid, alerting developers to potential tampering.
-
Regulatory Compliance: Laws such as the General Data Protection Regulation (GDPR) and California Consumer Privacy Act (CCPA) mandate encryption for handling sensitive user data.
-
Building Trust: Users are more likely to trust applications that prioritize their privacy and security. Encryption is a visible commitment to safeguarding their data.
Types of Encryption
Encryption can be broadly categorized into two types: symmetric and asymmetric. Each has its strengths, weaknesses, and use cases.
Symmetric Encryption
Symmetric encryption uses a single key for both encryption and decryption. It’s efficient and suitable for encrypting large amounts of data.
Examples of Symmetric Encryption Algorithms:
- Advanced Encryption Standard (AES):
- Widely used in applications, AES supports key lengths of 128, 192, or 256 bits for varying levels of security.
- It is considered highly secure and is the standard for encrypting sensitive data worldwide.
- Triple DES (3DES):
- An extension of the DES algorithm, 3DES applies the DES algorithm three times for added security.
- Though effective, it’s slower and being phased out in favor of AES.
Use Cases:
- Encrypting files or databases.
- Securing network communications (e.g., VPNs).
Asymmetric Encryption
Asymmetric encryption uses two keys: a public key for encryption and a private key for decryption. This approach eliminates the need to share secret keys, making it ideal for secure communications.
Examples of Asymmetric Encryption Algorithms:
- RSA (Rivest–Shamir–Adleman):
- RSA is commonly used for securing sensitive data like digital certificates and emails.
- It relies on the difficulty of factoring large prime numbers for security.
- Elliptic Curve Cryptography (ECC):
- ECC provides similar security to RSA but with smaller key sizes, making it faster and more efficient.
Use Cases:
- Digital signatures.
- Encrypting emails or session keys.
Common Encryption Techniques in Development
Hashing
Hashing is a one-way encryption technique that converts data into a fixed-size string of characters. It’s commonly used for storing passwords securely.
Key Algorithms:
- SHA-256 (Secure Hash Algorithm):
- A cryptographic hash function that produces a 256-bit hash, commonly used for password hashing and data integrity verification.
- BCrypt:
- A hashing function designed for securely storing passwords. It incorporates salting and is resistant to brute force attacks.
Example (Python using bcrypt):
import bcrypt
password = b"securepassword123"
hashed = bcrypt.hashpw(password, bcrypt.gensalt())
print(hashed)
End-to-End Encryption (E2EE)
E2EE ensures that only the sender and recipient can read a message, even if it’s intercepted during transmission. Popular applications like WhatsApp and Signal rely on E2EE for secure messaging.
How It Works:
- Messages are encrypted on the sender’s device and decrypted only on the recipient’s device.
- Even the service provider cannot access the plaintext messages.
TLS/SSL Encryption
Transport Layer Security (TLS) and its predecessor, Secure Sockets Layer (SSL), encrypt data in transit between clients and servers. This ensures confidentiality and integrity for web traffic.
Example Use Case: HTTPS uses TLS to secure web communications, protecting users from man-in-the-middle attacks.
How to Implement Encryption in Applications
- Choose the Right Algorithm:
- Evaluate your application’s needs (e.g., speed, security, compatibility) and select an appropriate encryption algorithm.
- Avoid Writing Your Own Encryption:
- Rely on established libraries like OpenSSL or PyCrypto instead of creating custom encryption algorithms, which are prone to vulnerabilities.
- Use Secure Key Management:
- Store keys securely using services like AWS Secrets Manager or HashiCorp Vault.
- Rotate keys periodically to minimize risks.
- Encrypt Sensitive Data Everywhere:
- Encrypt data at rest (e.g., databases, storage devices) and in transit (e.g., HTTPS, email encryption).
- Test Regularly:
- Conduct penetration testing to identify weaknesses in your encryption implementation.
- Use tools like Wireshark to ensure encrypted data cannot be easily intercepted.
Challenges in Encryption
Despite its benefits, encryption is not without challenges:
- Performance Overhead:
- Encrypting large amounts of data can slow down systems. Use optimized algorithms like AES-GCM for better performance.
- Key Management:
- Poor key storage or sharing practices can compromise the entire encryption process.
- Legal Restrictions:
- Some countries impose restrictions on the use and export of strong encryption algorithms.
Future Trends in Encryption
- Post-Quantum Cryptography:
- Quantum computers could potentially break current encryption algorithms like RSA. Researchers are developing quantum-resistant algorithms to address this threat.
- Homomorphic Encryption:
- This technique allows computations to be performed on encrypted data without decrypting it first, preserving privacy.
- Blockchain Integration:
- Blockchain technology is enhancing data security by combining encryption with decentralized ledgers.
Conclusion
Encryption is a fundamental tool for developers to protect user data, ensure application integrity, and comply with legal standards. By understanding how encryption works and implementing best practices, you can create secure applications that users trust.
Start integrating encryption into your projects today to enhance their security and resilience in an increasingly data-driven world.