Published
- 5 min read
Writing Test Cases for Security Vulnerabilities
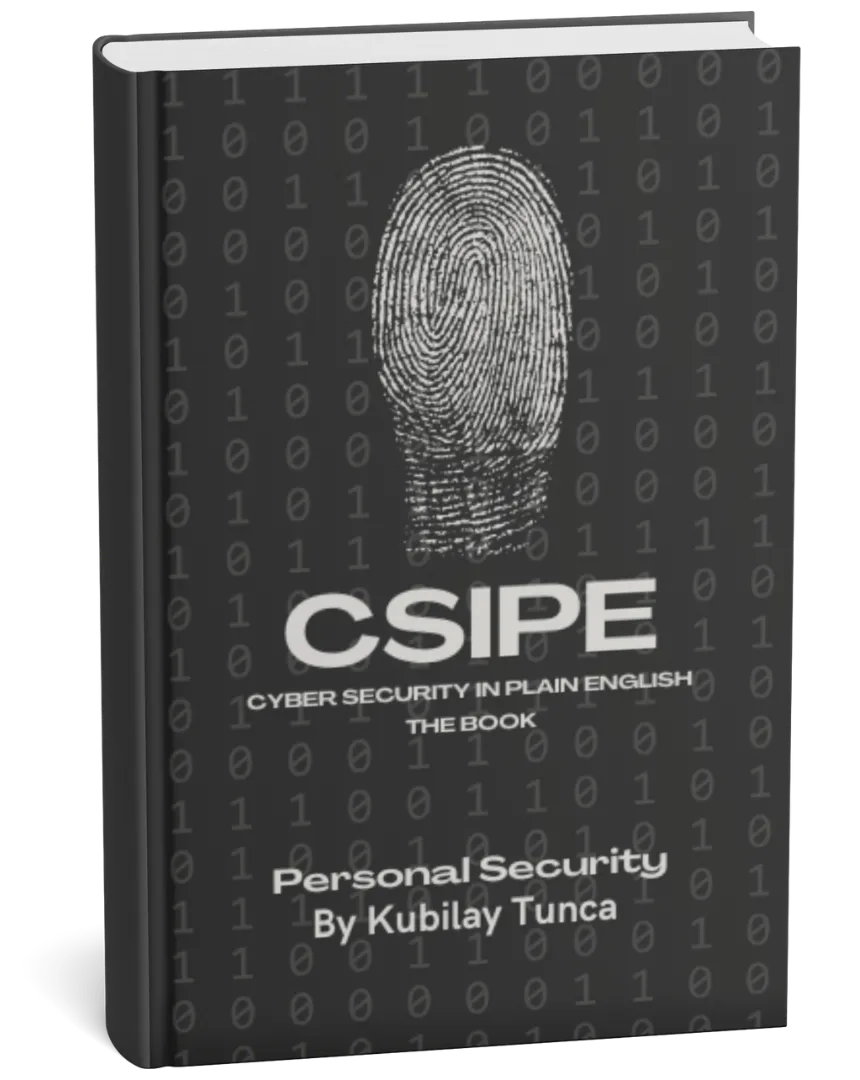
Protect Your Digital Life, Hassle-Free
Private Security in Plain English is your friendly guide to stopping common cyber threats—no tech degree required. Learn the simple, practical steps to safeguard your passwords, devices, and personal data in a language you’ll actually understand.
Buy the Ebook NowIntroduction
In software development, security vulnerabilities are among the most critical challenges to address. Writing effective test cases specifically aimed at identifying and mitigating these vulnerabilities ensures your application remains robust against attacks. By systematically testing for security flaws, developers can uncover weaknesses early in the development process, reducing the risk of exploitation and minimizing costly post-deployment fixes.
This comprehensive guide explores the methodology, tools, and strategies for writing test cases tailored to detect security vulnerabilities in your applications.
The Importance of Writing Security Test Cases
1. Proactive Vulnerability Detection
Security test cases enable developers to identify vulnerabilities during the development phase, minimizing the likelihood of security breaches.
2. Improved Code Quality
By testing for security issues, developers write more secure and maintainable code.
3. Compliance and Risk Management
Many industries require proof of security testing to meet regulatory standards such as GDPR, HIPAA, and PCI DSS.
4. Cost Efficiency
Fixing vulnerabilities during development is significantly less expensive than addressing them post-deployment.
Types of Security Vulnerabilities Addressed by Test Cases
1. Injection Flaws
- Examples: SQL Injection, Command Injection, NoSQL Injection.
- Test Case Objective: Ensure user inputs are properly sanitized to prevent malicious injections.
2. Authentication and Session Management Issues
- Examples: Weak passwords, session fixation, credential stuffing.
- Test Case Objective: Validate strong authentication mechanisms and secure session handling.
3. Cross-Site Scripting (XSS)
- Examples: Reflected XSS, Stored XSS, DOM-based XSS.
- Test Case Objective: Ensure user inputs do not execute scripts in the browser.
4. Access Control Weaknesses
- Examples: Privilege escalation, broken access control.
- Test Case Objective: Verify access controls enforce least privilege principles.
5. Data Exposure
- Examples: Sensitive information leakage, unencrypted data.
- Test Case Objective: Ensure data is encrypted and access is restricted.
Writing Effective Security Test Cases
Step 1: Understand Application Architecture
Before writing test cases, document the application’s architecture, data flows, and security requirements. Identify components such as:
- APIs and endpoints.
- Authentication systems.
- User inputs and outputs.
Step 2: Define Test Objectives
Establish clear goals for each test case, focusing on specific vulnerabilities and components.
Example Objective:
“Ensure all user inputs in the login form are sanitized to prevent SQL injection.”
Step 3: Choose the Right Testing Methodology
- Static Testing: Analyzes code without executing it (e.g., checking for hardcoded credentials).
- Dynamic Testing: Evaluates the application in a running state (e.g., simulating injection attacks).
Step 4: Write Test Case Descriptions
Include detailed steps, expected results, and acceptance criteria for each test case.
Example Test Case (SQL Injection):
- Input: Enter
"' OR '1'='1"
in the username field. - Action: Submit the login form.
- Expected Result: The application rejects the input and displays an error.
Common Test Cases for Security Vulnerabilities
1. SQL Injection
Test Case:
- Inject SQL payloads (
' OR 1=1; --
) into input fields. - Verify that the application prevents unauthorized database queries.
2. Cross-Site Scripting (XSS)
Test Case:
- Inject
<script>alert('XSS')</script>
into form fields. - Ensure the application escapes or removes the script tags.
3. Authentication
Test Case:
- Attempt login using weak passwords (e.g.,
123456
). - Confirm that the application enforces password complexity requirements.
4. Session Management
Test Case:
- Reuse a session token after logging out.
- Verify the application invalidates the token.
5. Access Control
Test Case:
- Access admin-only endpoints as a regular user.
- Ensure the application denies access.
Tools for Security Test Case Implementation
1. OWASP ZAP
- Automates testing for common vulnerabilities such as XSS and SQL injection.
Example Use Case:
Crawl an application to identify endpoints and simulate attacks.
2. Burp Suite
- Provides advanced tools for testing web application security.
Example Use Case:
Intercept and manipulate HTTP requests to test server responses.
3. Postman
- Tests API endpoints for vulnerabilities like missing authentication and input validation.
4. Fuzz Testing Tools
- Tools: AFL (American Fuzzy Lop), Peach Fuzzer.
- Purpose: Generate random inputs to uncover unexpected application behavior.
Best Practices for Writing Security Test Cases
1. Automate Where Possible
Use tools to automate repetitive test cases, ensuring consistency and efficiency.
2. Collaborate with Security Teams
Work closely with security experts to identify high-risk areas and develop targeted test cases.
3. Incorporate Threat Modeling
Leverage threat modeling techniques (e.g., STRIDE) to guide test case development.
4. Focus on Real-World Scenarios
Design test cases that mimic real-world attack patterns to ensure practical relevance.
5. Iterate and Update
Regularly review and update test cases to address new threats and application changes.
Challenges in Security Test Case Development
1. False Positives
Automated tools may flag non-issues, leading to unnecessary debugging.
Solution: Combine automated tests with manual validation.
2. Evolving Threats
New vulnerabilities emerge frequently, requiring constant adaptation.
Solution: Stay informed with resources like OWASP and CVE databases.
3. Limited Resources
Time and expertise constraints can hinder thorough testing.
Solution: Prioritize high-risk areas and gradually expand coverage.
Advanced Techniques for Security Test Case Development
1. Behavior-Driven Development (BDD) for Security
Define security scenarios using plain language and integrate them into automated tests.
Example (Cucumber Syntax):
Given I am on the login page
When I submit SQL injection payload
Then I should see an error message
2. Continuous Security Testing
Integrate security tests into CI/CD pipelines to catch vulnerabilities during development.
Example (GitHub Actions):
jobs:
security-testing:
runs-on: ubuntu-latest
steps:
- name: Run OWASP ZAP
run: zap-baseline.py -t http://example.com
3. Test-Driven Development (TDD) for Security
Write security tests before implementing features to ensure vulnerabilities are addressed upfront.
Conclusion
Writing test cases for security vulnerabilities is a proactive approach to ensuring application resilience against cyber threats. By systematically identifying risks, simulating attacks, and refining defenses, developers can build secure systems that inspire user trust. Leveraging tools, best practices, and collaborative efforts, security test cases become a cornerstone of modern software development.
Start enhancing your application’s security posture today by integrating targeted, effective test cases into your development lifecycle.