Published
- 5 min read
What Developers Can Learn from the OWASP Juice Shop
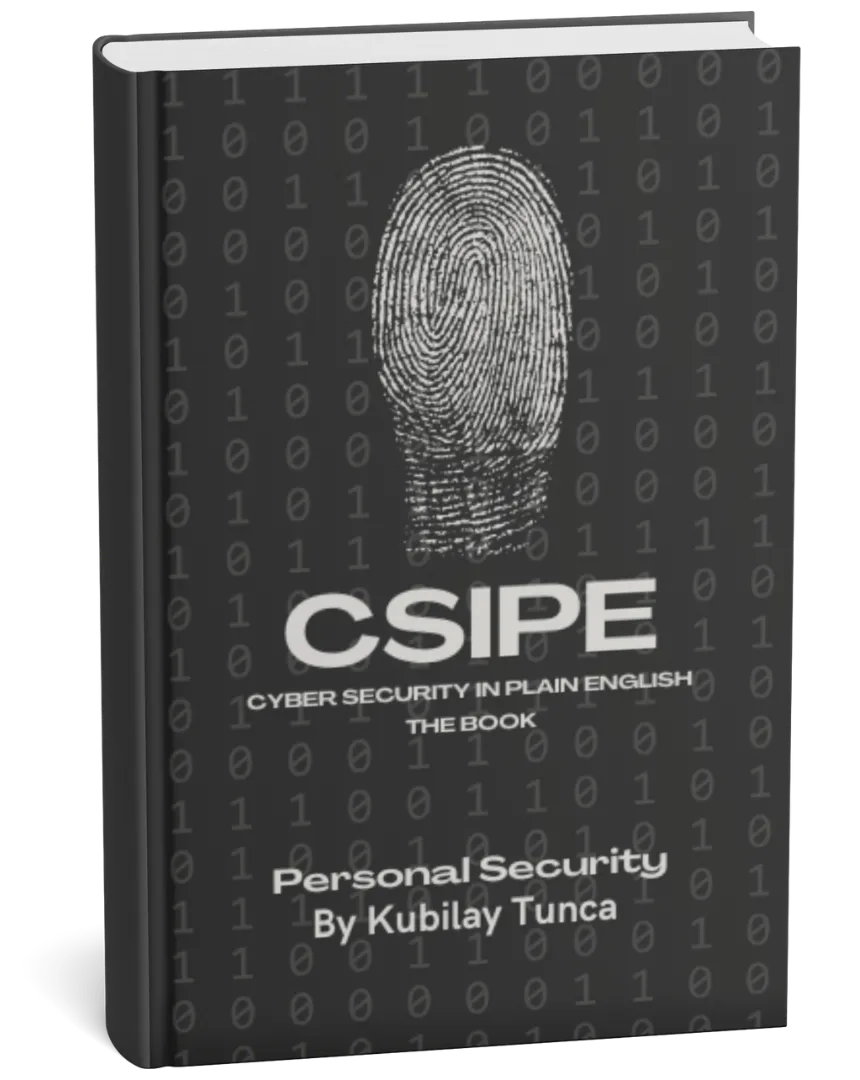
Protect Your Digital Life, Hassle-Free
Private Security in Plain English is your friendly guide to stopping common cyber threats—no tech degree required. Learn the simple, practical steps to safeguard your passwords, devices, and personal data in a language you’ll actually understand.
Buy the Ebook NowIntroduction
The OWASP Juice Shop is more than just a vulnerable web application—it’s a powerful educational tool designed to teach developers the importance of secure coding practices. By simulating real-world vulnerabilities, the Juice Shop provides hands-on experience in identifying, exploiting, and mitigating security flaws. This interactive approach makes it an invaluable resource for developers aiming to enhance their cybersecurity skills.
This article delves into the lessons developers can learn from the OWASP Juice Shop, its key features, and practical tips for applying these insights to build secure applications.
What is the OWASP Juice Shop?
The OWASP Juice Shop is an intentionally vulnerable web application that mimics a modern e-commerce platform. Developed as part of the Open Web Application Security Project (OWASP), it serves as a training ground for understanding and mitigating security vulnerabilities.
Key Features:
- Realistic Vulnerabilities: Simulates issues found in real-world applications, from SQL injection to Cross-Site Scripting (XSS).
- Gamified Challenges: Encourages users to find and exploit vulnerabilities, earning points for each challenge completed.
- Comprehensive Coverage: Includes vulnerabilities listed in the OWASP Top 10, such as Broken Authentication and Sensitive Data Exposure.
- Open Source: Freely available for anyone to use, customize, and contribute to.
Lessons Developers Can Learn from the OWASP Juice Shop
1. Understanding Common Vulnerabilities
The Juice Shop exposes developers to a wide range of security flaws, helping them recognize patterns and understand their root causes.
Example Vulnerabilities:
- SQL Injection: Exploit improperly sanitized inputs to manipulate database queries.
- Cross-Site Scripting (XSS): Inject malicious scripts into web pages viewed by other users.
- Insecure Direct Object References (IDOR): Access unauthorized data through predictable URLs.
Takeaway:
Familiarize yourself with these vulnerabilities and implement secure coding practices to prevent them.
2. Importance of Input Validation
One of the most common lessons in the Juice Shop is the critical role of input validation in preventing attacks.
Vulnerability Example:
- A search bar that accepts unvalidated input can lead to SQL injection.
Best Practices:
- Validate all user inputs on both the client and server side.
- Use parameterized queries or prepared statements to secure database interactions.
Code Example (Parameterized Query in Python):
import sqlite3
connection = sqlite3.connect("example.db")
cursor = connection.cursor()
query = "SELECT * FROM users WHERE username = ?"
cursor.execute(query, (user_input,))
3. The Value of Secure Authentication
The Juice Shop highlights the dangers of weak authentication mechanisms, such as predictable passwords and insecure session management.
Vulnerability Example:
- Using default credentials like “admin/admin” allows attackers to gain unauthorized access.
Best Practices:
- Implement strong password policies and enforce multi-factor authentication (MFA).
- Secure session cookies with the HttpOnly and Secure flags.
4. Implementing Proper Access Controls
By exploring vulnerabilities like IDOR, developers learn the importance of restricting access to sensitive resources.
Vulnerability Example:
- Modifying a user ID in a URL to access another user’s account.
Best Practices:
- Use role-based access control (RBAC) to define and enforce permissions.
- Validate authorization at every access point.
5. Encrypting Sensitive Data
The Juice Shop demonstrates how unencrypted sensitive data can be easily intercepted or stolen.
Vulnerability Example:
- Storing plaintext passwords in the database.
Best Practices:
- Hash passwords using algorithms like bcrypt or Argon2.
- Encrypt sensitive data at rest using AES-256.
Code Example (Password Hashing with bcrypt):
from bcrypt import hashpw, gensalt
password = "securepassword"
hashed_password = hashpw(password.encode(), gensalt())
How to Use the OWASP Juice Shop Effectively
1. Set Up a Local Environment
Run the Juice Shop locally using Docker, Node.js, or cloud platforms to create a controlled learning environment.
2. Start with Basic Challenges
Begin with easier challenges to build confidence and gradually progress to more complex vulnerabilities.
3. Document Your Findings
Keep detailed notes on each vulnerability, including how it was exploited and the steps needed to mitigate it.
4. Apply Lessons to Real Projects
Translate the knowledge gained from the Juice Shop into secure coding practices in your own applications.
Tools and Resources Complementing the OWASP Juice Shop
1. Burp Suite
A comprehensive tool for web application security testing. Use it alongside the Juice Shop to analyze vulnerabilities.
2. OWASP ZAP
An open-source security scanner that integrates seamlessly with the Juice Shop.
3. Secure Code Warrior
Offers gamified secure coding challenges to reinforce the lessons learned from the Juice Shop.
4. OWASP Cheat Sheets
Provides detailed guidelines for implementing secure practices.
Challenges and Common Pitfalls
1. Overlooking Logging and Monitoring
Without proper logging, detecting and responding to vulnerabilities becomes difficult.
Solution:
- Implement logging tools and monitor application activity for anomalies.
2. Underestimating Client-Side Security
Relying solely on server-side validation leaves client-side vulnerabilities exposed.
Solution:
- Secure both client-side and server-side components of your application.
3. Neglecting Regular Updates
Vulnerabilities evolve, and so do their mitigations. Failing to update the Juice Shop or your security knowledge limits its effectiveness.
Solution:
- Regularly update your tools and stay informed about new vulnerabilities.
Real-World Applications of Juice Shop Lessons
Scenario 1: Securing an E-Commerce Platform
- Problem: SQL injection in the product search feature.
- Solution: Implement parameterized queries and input validation, learned from the Juice Shop.
Scenario 2: Preventing XSS in a Blog Application
- Problem: User-submitted comments contained malicious scripts.
- Solution: Sanitize all inputs and encode outputs to neutralize script injection.
Scenario 3: Protecting User Accounts
- Problem: Weak password policies led to account compromise.
- Solution: Enforce strong passwords and implement MFA.
Conclusion
The OWASP Juice Shop is a treasure trove of knowledge for developers looking to enhance their secure coding skills. By exploring its vulnerabilities and challenges, developers can gain hands-on experience with real-world security issues and learn how to address them effectively.
Start your journey with the OWASP Juice Shop today and transform your applications into robust, secure systems that stand the test of time.