Published
- 10 min read
Step-by-Step: How to Encrypt Data in Python
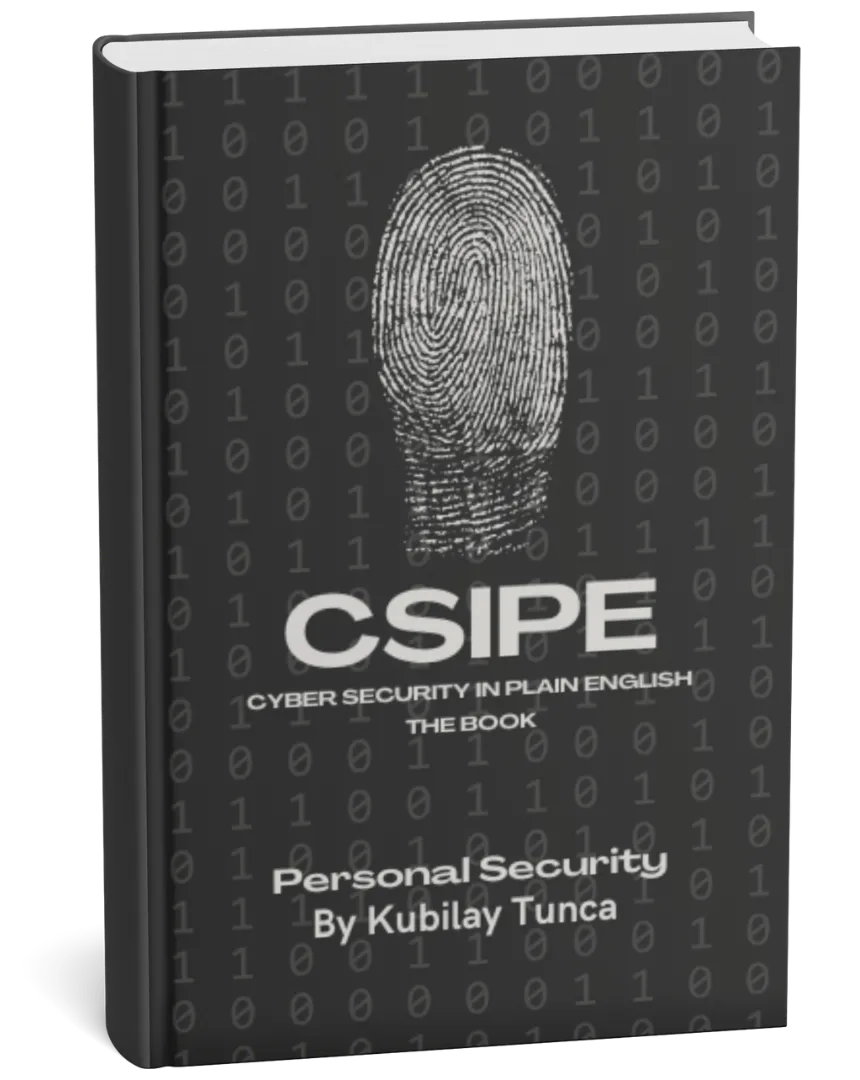
Protect Your Digital Life, Hassle-Free
Private Security in Plain English is your friendly guide to stopping common cyber threats—no tech degree required. Learn the simple, practical steps to safeguard your passwords, devices, and personal data in a language you’ll actually understand.
Buy the Ebook NowStep-by-Step: How to Encrypt Data in Python
In the digital age, protecting sensitive data is a critical responsibility for developers and organizations alike. Whether you’re handling customer information, proprietary business logic, or personal user credentials, encryption acts as a frontline defense against unauthorized access and data breaches. Python, with its rich ecosystem of libraries, makes it easier than ever to implement strong encryption techniques. However, navigating the world of cryptography can be daunting if you’re just getting started.
This comprehensive, step-by-step guide aims to demystify data encryption in Python. We will walk through the core concepts, explore common algorithms and best practices, and provide hands-on examples that illustrate exactly how to implement encryption in your projects. By the end, you’ll have the confidence to integrate secure encryption into your applications, bolstering your data protection strategy and aligning your code with industry standards.
Table of Contents
- Symmetric vs. Asymmetric Encryption
- Common Algorithms (AES, RSA, etc.)
- Keys, IVs, and Salts Explained
- Hashing vs. Encryption vs. Encoding
- Generating RSA Keys
- Encrypting and Decrypting with RSA
- Combining Asymmetric and Symmetric Techniques
- Encrypting Files Before Storage
- Sending Encrypted Messages Over a Network
- Encrypting Data in Databases
- Working with Cloud KMS (Key Management Services)
Why Encrypt Data? Understanding the Importance
Data encryption transforms readable information (plaintext) into an unreadable format (ciphertext) that can only be deciphered by those who possess the correct key. This measure is crucial for safeguarding:
- User Credentials: Passwords, API keys, and tokens must be protected from unauthorized access.
- Personal Identifiable Information (PII): Names, addresses, credit card details, and other sensitive personal data.
- Intellectual Property: Proprietary algorithms, product roadmaps, and business strategies.
When security incidents like breaches or insider threats occur, encrypted data mitigates potential damage. Attackers who gain access to encrypted data face a steep challenge in turning that gibberish back into meaningful information. Encryption is a cornerstone of privacy, trust, and compliance with regulations like GDPR, HIPAA, and PCI DSS.
Core Concepts in Encryption
Symmetric vs. Asymmetric Encryption
Symmetric Encryption:
- Uses the same key for encryption and decryption.
- Typically faster and more suitable for large volumes of data.
- Common symmetric algorithms: AES (Advanced Encryption Standard), ChaCha20.
Asymmetric Encryption:
- Uses a pair of keys: a public key for encryption and a private key for decryption.
- Often employed for key exchange, digital signatures, and scenarios where securely sharing a single key is challenging.
- Common asymmetric algorithms: RSA, Elliptic Curve Cryptography (ECC).
Quick Tip:
- Symmetric = Shared Secret Key
- Asymmetric = Key Pair (Public/Private)
Common Algorithms (AES, RSA, etc.)
-
AES (Advanced Encryption Standard): A widely used symmetric cipher for its strength and speed. Supports key sizes of 128, 192, and 256 bits.
-
RSA: A popular asymmetric algorithm used for secure key exchanges, digital signatures, and encrypting small amounts of data (like symmetric keys).
-
Elliptic Curve Cryptography (ECC): Offers similar or better security than RSA with smaller keys, often used in modern systems requiring efficient cryptography.
Keys, IVs, and Salts Explained
-
Key: A secret piece of data used by encryption algorithms. Must be kept confidential.
-
IV (Initialization Vector): A random or pseudo-random input to encryption functions to ensure distinct ciphertexts even if the same data and key are reused.
-
Salt: Used in hashing and key derivation functions to prevent attacks like rainbow table lookups. Salts ensure the same password doesn’t always produce the same hash.
Hashing vs. Encryption vs. Encoding
-
Hashing: One-way transformation. You cannot retrieve the original data from the hash. Used for storing passwords.
-
Encryption: Two-way transformation. With the correct key, you can decrypt ciphertext back into plaintext.
-
Encoding: Not a security measure. Converting data into a different format (like Base64) for transport or readability.
Choosing the Right Python Libraries
The cryptography
Library
Cryptography is a well-maintained, actively developed Python library for secure cryptographic operations. It offers:
- High-level recipes (Fernet) for symmetric encryption.
- Low-level primitives for implementing AES, RSA, and more.
- Well-reviewed and secure code built on top of OpenSSL.
Why Use cryptography
?
- Actively maintained by experts.
- Easy-to-use high-level APIs.
- Broad functionality covering multiple cryptographic needs.
PyNaCl and Others
PyNaCl provides bindings to the libsodium library. It offers high-level functions for encryption, signatures, and key exchange, focusing on modern, secure defaults like Curve25519.
Other specialized libraries exist for niche use cases, but cryptography
and PyNaCl are common starting points.
Built-in Modules vs. Third-Party Solutions
Python’s standard library includes hashlib
and hmac
for hashing and message authentication, but it does not include modern encryption algorithms out-of-the-box. Relying on cryptography
or PyNaCl is recommended to ensure you have secure, maintained primitives.
Setting Up Your Environment
Installing Dependencies
Assuming you have Python 3 installed, you can install cryptography
with:
pip install cryptography
For PyNaCl:
pip install pynacl
Creating a Secure Development Process
- Version Control: Keep keys out of source control. Use environment variables or configuration files excluded from git.
- Testing & CI: Integrate tests that confirm encryption and decryption work as expected.
- Security Reviews: Periodically review your cryptographic code and dependencies for updates or advisories.
- Step-by-Step Example: Symmetric Encryption with Fernet (AES)
The cryptography.fernet module provides a simple interface for symmetric encryption with AES in CBC mode, plus HMAC authentication. Fernet handles:
- Key generation
- Encryption and decryption
- Integrity checking of messages
- Generating and Managing Keys
Steps:
Import Fernet:
from cryptography.fernet import Fernet
Generate a Key:
key = Fernet.generate_key()
Store the Key Securely:
Save the key in a secure location, such as a locked-down file or an environment variable. Do Not hard-code keys in your source code or repository.
Example:
from cryptography.fernet import Fernet
key = Fernet.generate_key()
with open("secret.key", "wb") as key_file:
key_file.write(key)
Encrypting Data Steps:
Load the Key:
with open("secret.key", "rb") as key_file:
key = key_file.read()
Create a Fernet Instance:
f = Fernet(key)
Encrypt:
plaintext = b"Sensitive data here"
ciphertext = f.encrypt(plaintext)
print(ciphertext) # This will look like a long base64-encoded string
Decrypting Data Steps:
Load the Same Key:
with open("secret.key", "rb") as key_file:
key = key_file.read()
f = Fernet(key)
Decrypt:
decrypted_data = f.decrypt(ciphertext)
print(decrypted_data) # b"Sensitive data here"
Handling Errors and Exceptions
If the ciphertext is tampered with or the key is incorrect, f.decrypt() will raise cryptography.fernet.InvalidToken.
Example:
try:
plaintext = f.decrypt(ciphertext)
except cryptography.fernet.InvalidToken:
print("Error: Invalid Key or corrupted ciphertext!")
Advanced Topics in Symmetric Encryption
Fernet provides authenticated encryption, which ensures data integrity. However, you might need more control or additional features.
Using AES GCM Mode for Authenticated Encryption AES-GCM mode provides both encryption and authenticity in one step. It’s faster and widely recommended.
Example with AES GCM:
from cryptography.hazmat.primitives.ciphers.aead import AESGCM
import os
key = AESGCM.generate_key(bit_length=256)
aesgcm = AESGCM(key)
nonce = os.urandom(12) # GCM recommended nonce size is 96 bits
data = b"Highly confidential message"
aad = b"Associated data" # can be empty if not needed
ciphertext = aesgcm.encrypt(nonce, data, aad)
plaintext = aesgcm.decrypt(nonce, ciphertext, aad)
Storing and Rotating Keys Securely
Best practices:
Store Keys in a Secure Vault: Use services like HashiCorp Vault or AWS KMS. Rotate Keys Regularly: Periodic rotation reduces the impact of key compromise. Use a Key Derivation Function (KDF): If deriving keys from passwords, use PBKDF2, scrypt, or Argon2 to slow down brute-force attacks. Example (PBKDF2):
from cryptography.hazmat.primitives.kdf.pbkdf2 import PBKDF2HMAC
from cryptography.hazmat.primitives import hashes
from base64 import urlsafe_b64encode
password = b"mysupersecretpassword"
salt = os.urandom(16)
kdf = PBKDF2HMAC(
algorithm=hashes.SHA256(),
length=32,
salt=salt,
iterations=100000,
)
key = urlsafe_b64encode(kdf.derive(password))
Asymmetric Encryption and Key Management
Asymmetric encryption can be used to share symmetric keys securely over an untrusted channel. The recipient’s public key encrypts the data, and only their private key can decrypt it.
Generating RSA Keys
Using cryptography to generate RSA keys:
from cryptography.hazmat.primitives.asymmetric import rsa
private_key = rsa.generate_private_key(
public_exponent=65537,
key_size=2048,
)
public_key = private_key.public_key()
Encrypting and Decrypting with RSA
RSA is typically used to encrypt small pieces of data or symmetric keys, not large files, due to performance reasons.
Encryption:
from cryptography.hazmat.primitives.asymmetric import padding
from cryptography.hazmat.primitives import hashes
message = b"Symmetric key or small secret"
ciphertext = public_key.encrypt(
message,
padding.OAEP(
mgf=padding.MGF1(algorithm=hashes.SHA256()),
algorithm=hashes.SHA256(),
label=None
)
)
Decryption:
plaintext = private_key.decrypt(
ciphertext,
padding.OAEP(
mgf=padding.MGF1(algorithm=hashes.SHA256()),
algorithm=hashes.SHA256(),
label=None
)
)
Combining Asymmetric and Symmetric Techniques
A common pattern:
Generate a symmetric key for encrypting large data. Encrypt that symmetric key with the recipient’s public RSA key. Send the encrypted symmetric key + encrypted data. Recipient uses their private RSA key to decrypt the symmetric key, then decrypts the data. This approach leverages the performance of symmetric encryption with the key distribution benefits of asymmetric encryption.
Practical Use Cases and Patterns
Encrypting Files Before Storage
Example:
- Read a file’s contents into memory.
- Use Fernet or AES GCM to encrypt the contents.
- Write the encrypted data back to disk.
- Store the encryption key in a secure store or environment variable.
with open("myfile.txt", "rb") as f:
data = f.read()
ciphertext = f.encrypt(data)
with open("myfile.enc", "wb") as f:
f.write(ciphertext)
Sending Encrypted Messages Over a Network
- Generate a session key (symmetric).
- Encrypt the session key with the recipient’s public RSA key.
- Send the encrypted session key and the ciphertext message.
- The recipient decrypts the session key and then decrypts the message.
Encrypting Data in Databases
- Encrypt sensitive fields (like credit card numbers) before inserting into the database.
- Store the encrypted data as a binary or base64 field.
- Decrypt when needed using the correct key.
Working with Cloud KMS (Key Management Services)
- Leverage AWS KMS, Google KMS, and Azure Key Vault to handle keys for you.
- Request a data key from the KMS, encrypt data locally, and discard the key after use.
- Benefit: Reduces the risk of key exposure and simplifies rotation.
Performance Considerations and Optimization
- Symmetric encryption is fast: Using AES with hardware acceleration is often sufficient.
- Minimize Data Transfers: Encrypt data once and store it encrypted to avoid multiple encryption/decryption cycles.
- Profile Your Code: If encryption is a bottleneck, optimize I/O or use streaming modes for large files.
Testing and Validating Your Encryption Implementation
Unit and Integration Tests
Recommended Tests:
- Encrypt/Decrypt Round Trip: Encrypt test data and verify that decrypting returns the original.
- Tampered Ciphertext: Modify ciphertext slightly and ensure decryption fails.
- Key Rotation Test: Verify that after rotating keys, old ciphertexts can still be decrypted if keys are available.
Penetration Testing and Audits
- Hire security professionals to test your implementation.
- Use tools that scan for known cryptographic weaknesses.
- Keep dependencies updated to avoid vulnerabilities in underlying libraries.
Common Pitfalls and How to Avoid Them
Hardcoding Keys:
- Bad: Storing keys in the source code.
- Solution: Use environment variables, configuration management, or a secrets manager.
Weak Keys or Passwords:
- Bad: Using short keys or predictable passwords.
- Solution: Use strong random keys, password managers, or KDFs.
Ignoring Integrity Checks:
- Bad: Not verifying that ciphertext is unmodified.
- Solution: Use authenticated encryption like AES GCM or Fernet.
Lack of Documentation and Auditing:
- Bad: Future developers don’t know how or why encryption was implemented.
- Solution: Document key paths, algorithms, and policies.
Maintaining and Updating Your Encryption Schemes
Algorithm Upgrades:
- If AES-256 or RSA-2048 becomes insufficient (rare but possible), have a plan for re-encrypting data with stronger keys.
Key Rotation Schedules:
- Rotate keys at set intervals or after security incidents.
- Update systems to handle multiple active keys gracefully.
Regular Audits:
- Periodically review cryptographic code and configurations.
- Check for updated best practices or deprecation notices in chosen libraries.
Conclusion
Implementing encryption in Python doesn’t have to be daunting. By understanding symmetric and asymmetric cryptography, choosing the right libraries, and following best practices for key management and authenticated encryption, you can secure your data effectively.
Key Takeaways:
- Start with Symmetric Encryption (Fernet/AES): Simpler and sufficient for most data-at-rest scenarios.
- Use Asymmetric Encryption Sparingly: Mainly for secure key exchange, not bulk data encryption.
- Document and Test: Clear documentation and thorough tests ensure your encryption works as intended.
- Be Adaptive: Stay current with evolving cryptographic standards, rotate keys periodically, and maintain a security-conscious culture.
By following these steps and using the provided code samples, you’ll ensure that sensitive information in your Python applications remains private, intact, and protected against unauthorized access.