Published
- 5 min read
How to Implement Secure Error Handling
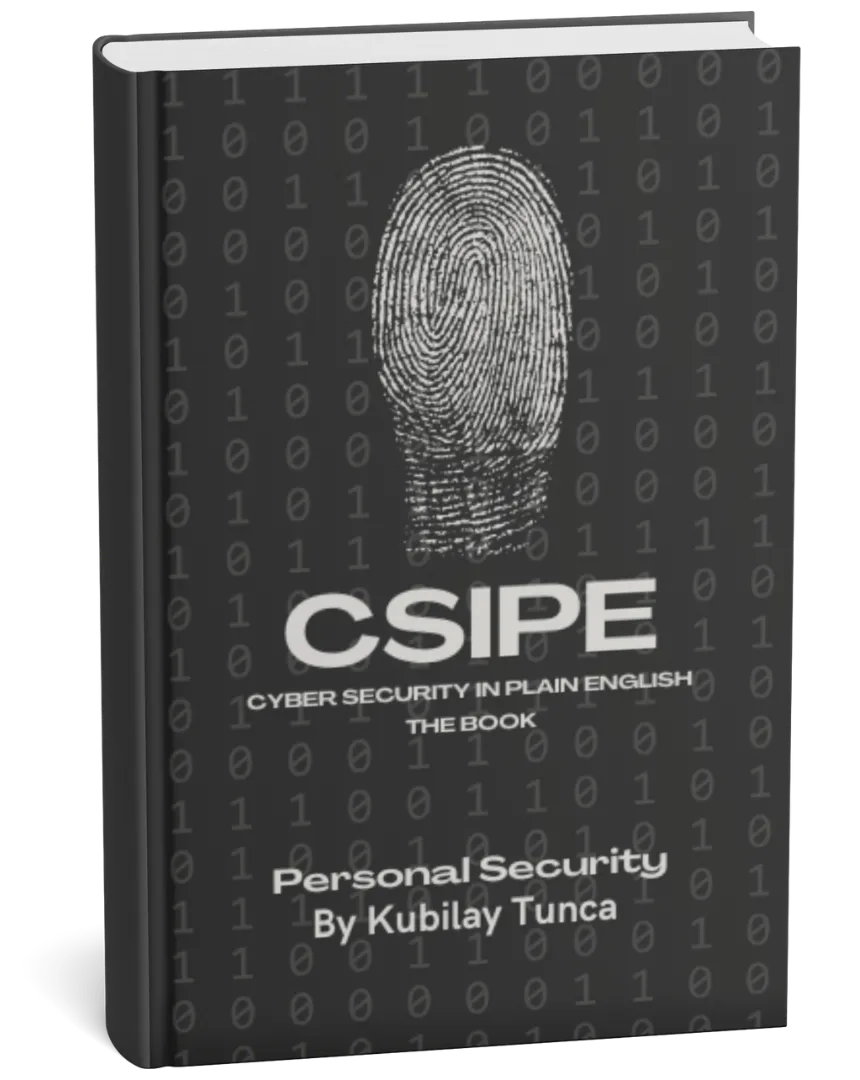
Protect Your Digital Life, Hassle-Free
Private Security in Plain English is your friendly guide to stopping common cyber threats—no tech degree required. Learn the simple, practical steps to safeguard your passwords, devices, and personal data in a language you’ll actually understand.
Buy the Ebook NowIntroduction
Error handling is an essential part of software development, ensuring that applications respond gracefully to unexpected situations. However, improper error handling can inadvertently expose sensitive information, providing attackers with valuable insights into an application’s inner workings. Secure error handling addresses these risks by concealing critical details while maintaining functionality and user experience.
This article explores the principles and techniques of secure error handling, offering practical guidance for developers to implement error management strategies that protect their applications and users from potential threats.
Why Secure Error Handling Matters
Errors are inevitable in any application. They can arise from user input, system failures, or external dependencies. While handling errors is a standard development practice, doing so insecurely can lead to vulnerabilities, such as:
- Information Disclosure:
- Detailed error messages may reveal sensitive information, such as database structures, server configurations, or API endpoints.
- Attack Facilitation:
- Exposed stack traces or debug information can help attackers identify vulnerabilities to exploit.
- User Confusion:
- Poorly designed error messages can confuse users and reduce trust in the application.
Secure error handling minimizes these risks by controlling the information exposed to users and attackers while providing developers with sufficient data to diagnose and fix issues.
Principles of Secure Error Handling
Secure error handling is guided by several key principles that ensure errors are managed effectively without compromising security.
1. Limit Information Disclosure
Expose only the information necessary for users to understand the error. Avoid technical details or stack traces, which can provide attackers with valuable insights.
- Good Example: “An error occurred while processing your request. Please try again later.”
- Bad Example: “SQL Error: Invalid syntax near ‘WHERE’ in query ‘SELECT * FROM users WHERE id = ?‘“
2. Log Detailed Errors Internally
While user-facing error messages should be generic, detailed logs are essential for developers and administrators. These logs should include information about the error, its context, and steps to reproduce it.
3. Use Centralized Error Handling
Centralized error handling ensures consistent practices across the application. It also simplifies updates and ensures compliance with security standards.
4. Avoid Revealing Business Logic
Error messages should not disclose details about your application’s business logic or workflows. For example, avoid messages like “User ID not found” and instead use a generic response like “Invalid credentials.”
5. Validate and Sanitize Inputs
Many errors stem from unexpected or malicious inputs. Validate and sanitize all inputs to prevent errors that could be exploited, such as SQL injection or buffer overflows.
Techniques for Implementing Secure Error Handling
1. Design User-Friendly Error Messages
User-facing error messages should be clear, concise, and non-technical. They should guide users on what to do next without exposing internal details.
Example:
Instead of “NullPointerException: object reference is null,” use: “We encountered an issue while processing your request. Please try again.”
2. Log Errors Securely
Error logs should provide sufficient detail for debugging but must be secured to prevent unauthorized access. Logs should also exclude sensitive data, such as user passwords or personal information.
Best Practices for Logging:
- Use unique identifiers for errors to correlate them with logs without exposing details.
- Store logs in secure locations, such as encrypted log files or centralized logging systems.
- Monitor logs for patterns that indicate potential attacks.
3. Implement Error Codes
Error codes help standardize error handling and simplify troubleshooting. For example, HTTP status codes like 400 (Bad Request) or 500 (Internal Server Error) convey the nature of the problem without revealing specifics.
Example:
- E001: “Invalid input provided.”
- E500: “Unexpected server error.”
4. Catch and Handle Exceptions
Proper exception handling ensures that errors do not propagate to users or other parts of the application. Catch exceptions at appropriate levels and use fallback mechanisms to maintain functionality.
Example (Python):
try:
result = process_request()
except ValueError as e:
log_error(e)
return "Invalid input."
except Exception as e:
log_error(e)
return "An unexpected error occurred."
5. Protect Error Pages
For web applications, error pages often expose details that can aid attackers. Customize error pages to display generic messages and avoid default server-generated responses.
Example:
- Replace the default “500 Internal Server Error” page with a custom page that says: “We’re experiencing technical difficulties. Please try again later.”
6. Use Secure Debugging Practices
Debugging is essential during development, but exposing debug information in production is a security risk. Ensure that debug modes are disabled in production environments and that debug logs are secured.
Tools for Secure Error Handling
Several tools and frameworks can help developers implement secure error handling practices:
Logging Tools
- ELK Stack (Elasticsearch, Logstash, Kibana): For centralized logging and analysis.
- Splunk: For real-time log monitoring and security insights.
Error Tracking Tools
- Sentry: Tracks errors and exceptions with detailed context.
- Bugsnag: Provides real-time error monitoring and diagnostics.
Framework-Specific Tools
- Django (Python):
- Django’s
DEBUG
setting should be set toFalse
in production. - Use middleware to handle errors consistently.
- Spring Boot (Java):
- Use
@ControllerAdvice
for centralized exception handling. - Configure custom error pages for HTTP errors.
Integrating Secure Error Handling into Your Workflow
Secure error handling should be an integral part of your development and deployment processes. Here’s how to achieve this:
-
Establish Guidelines: Define clear standards for user-facing messages, logging practices, and exception handling.
-
Review and Test: Conduct code reviews to identify insecure error handling practices. Use automated tests to verify that errors are handled securely and gracefully.
-
Monitor and Improve: Continuously monitor logs and error metrics to identify patterns and improve your error handling strategies.
Conclusion
Secure error handling is a critical component of application security, preventing sensitive information from being exposed while ensuring a positive user experience. By following the principles and techniques outlined in this article, developers can protect their applications from potential threats and improve their overall reliability.
Start implementing secure error handling practices today to strengthen your application’s defenses and build user trust. A secure application is not only functional but also resilient in the face of unexpected challenges.