Published
- 6 min read
Best Practices for Writing Secure Code
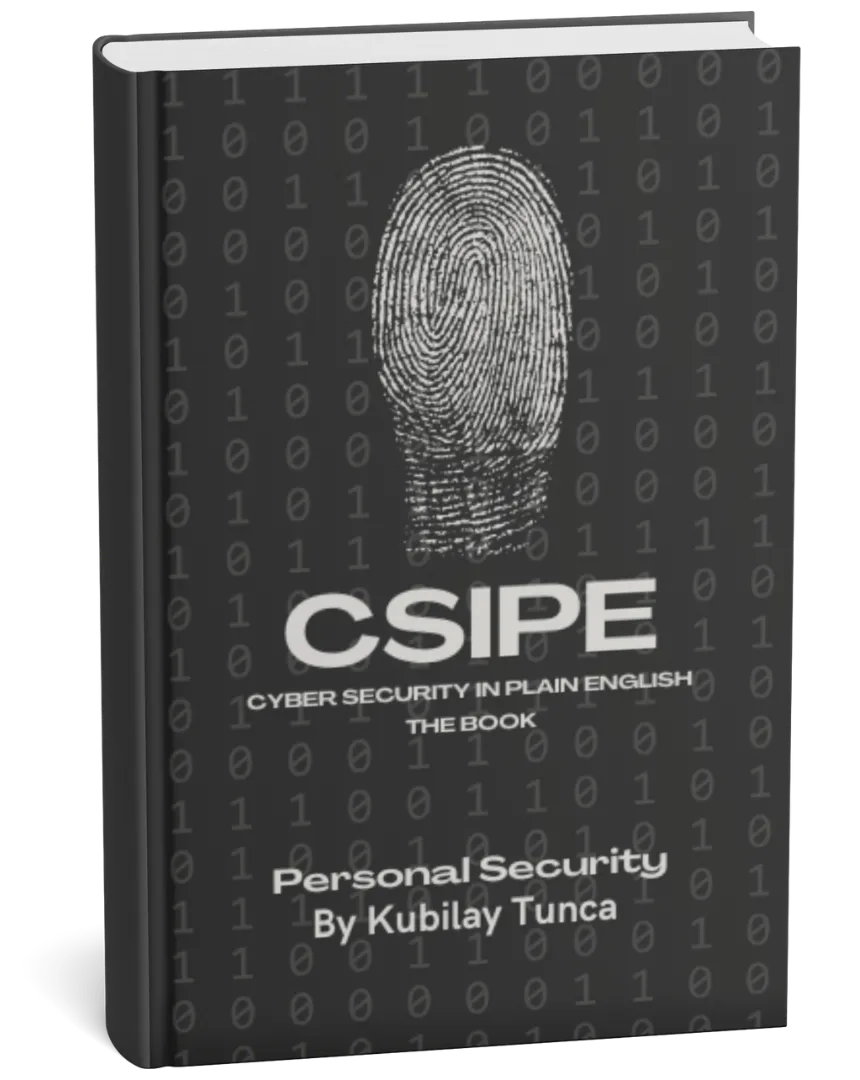
Protect Your Digital Life, Hassle-Free
Private Security in Plain English is your friendly guide to stopping common cyber threats—no tech degree required. Learn the simple, practical steps to safeguard your passwords, devices, and personal data in a language you’ll actually understand.
Buy the Ebook NowIntroduction
Writing secure code is no longer optional in the development process—it is a critical skill that every developer must master. As cyber threats evolve in complexity and scale, the cost of failing to address vulnerabilities in your code can lead to devastating consequences, including data breaches, legal liabilities, and a loss of user trust. Secure coding practices not only mitigate these risks but also contribute to building resilient, high-quality applications.
This article provides a comprehensive exploration of best practices for writing secure code. It delves into key principles, common pitfalls, and actionable strategies that developers can implement to ensure their applications are robust against potential vulnerabilities. Whether you’re an experienced developer or just beginning your journey in secure coding, this guide will equip you with the knowledge and tools to safeguard your applications.
Why Secure Coding Matters
The digital age has brought unprecedented connectivity and convenience, but it has also introduced a host of security challenges. Every application, regardless of its scale or purpose, is a potential target for malicious actors seeking to exploit vulnerabilities for personal or financial gain. Secure coding addresses these risks at their root, ensuring that your application is built on a foundation of security.
Insecure code can lead to vulnerabilities such as injection attacks, broken authentication, and data exposure. For example, a single SQL injection flaw can allow attackers to manipulate a database, exposing or corrupting sensitive information. By adopting secure coding practices, developers can preemptively address these risks and create systems that are resilient to attacks.
Furthermore, regulatory frameworks such as GDPR, HIPAA, and PCI DSS require organizations to implement security measures in their software development processes. Failure to comply can result in severe penalties, making secure coding not just a best practice but a legal necessity.
Principles of Secure Coding
Secure coding is guided by several core principles that help developers create reliable and protected applications. These principles are universally applicable across programming languages and development frameworks.
Principle of Least Privilege
The principle of least privilege dictates that code should operate with the minimum permissions necessary to perform its functions. For example, a database query module should only have access to the tables it needs, rather than full administrative privileges. By limiting access, you reduce the potential damage in the event of a compromise.
Defense in Depth
Defense in depth involves implementing multiple layers of security to protect your application. This approach ensures that even if one security measure fails, others remain in place to thwart an attack. For instance, input validation, parameterized queries, and encryption can work together to prevent SQL injection attacks.
Secure by Default
Applications should be secure out of the box. This means that default configurations should prioritize security, such as enforcing strong password requirements or enabling secure communication protocols like HTTPS.
Fail Securely
When errors occur, your application should fail in a way that does not compromise security. For example, if a user authentication system encounters an error, it should not grant access by default. Instead, it should deny access until the issue is resolved.
Avoid Security Through Obscurity
Relying on secrecy alone to protect your application is a flawed strategy. While it’s acceptable to keep certain implementation details private, your primary security measures should not depend on attackers remaining unaware of your methods. Instead, rely on robust and tested security practices.
Secure Coding Techniques
Developers can incorporate various techniques into their workflow to write secure code. These techniques address different aspects of the development process, from input validation to error handling.
Input Validation
Input validation is a cornerstone of secure coding. It involves verifying that user inputs conform to expected formats and rejecting anything that deviates. For example, if a form field expects a numeric value, ensure that only numeric input is accepted.
Proper input validation prevents many types of attacks, including SQL injection, cross-site scripting (XSS), and buffer overflows. Use whitelists to define acceptable input ranges or formats, as blacklists can be incomplete and easily bypassed.
Output Encoding
Output encoding ensures that user-generated content is displayed as plain text, rather than executable code. This is particularly important for web applications that handle HTML, as improper encoding can lead to XSS vulnerabilities. Libraries like OWASP’s ESAPI can simplify this process.
Secure Password Storage
Passwords should never be stored in plaintext. Instead, they should be hashed using secure algorithms such as bcrypt, Argon2, or PBKDF2. Hashing converts passwords into a fixed-length representation, which cannot be reversed. Additionally, salt should be added to hashes to make them resistant to rainbow table attacks.
Use Parameterized Queries
Parameterized queries, also known as prepared statements, prevent SQL injection attacks by separating query structure from user input. This ensures that user-provided data is treated as values rather than executable commands.
For example, in Python with SQLAlchemy:
query = "SELECT * FROM users WHERE id = :user_id"
db.execute(query, {"user_id": user_input})
Implement Secure APIs
APIs are often targeted by attackers, making their security a top priority. Use authentication and authorization mechanisms such as OAuth 2.0 to protect APIs. Additionally, validate all incoming data, enforce rate limiting, and use HTTPS to encrypt communication.
Common Pitfalls in Secure Coding
Even experienced developers can inadvertently introduce vulnerabilities into their code. Awareness of these common pitfalls can help you avoid them.
Hardcoding Secrets
Hardcoding sensitive information like API keys, credentials, or encryption keys into your code is a critical mistake. These secrets can be easily extracted, especially if the code is shared or deployed publicly. Instead, use secure storage solutions like AWS Secrets Manager or HashiCorp Vault.
Inadequate Error Handling
Error messages should not expose sensitive details about your application’s internal workings. For instance, a database error message that reveals table names or query structures can provide attackers with valuable information. Always log detailed errors for internal use, but display generic messages to users.
Overlooking Dependency Risks
Third-party libraries and frameworks can introduce vulnerabilities if not properly managed. Regularly update your dependencies and use tools like Snyk or OWASP Dependency-Check to identify and remediate security risks.
Tools for Secure Development
Several tools can assist developers in writing secure code and identifying vulnerabilities. These tools integrate seamlessly into development workflows and automate many aspects of security testing.
- Static Application Security Testing (SAST): Tools like SonarQube analyze source code to identify vulnerabilities without executing it.
- Dynamic Application Security Testing (DAST): Tools like Burp Suite simulate attacks on a running application to uncover weaknesses.
- Dependency Scanners: Snyk and Dependabot help manage third-party risks by identifying vulnerabilities in dependencies.
Building a Security-First Culture
Secure coding is not an individual effort—it requires a collective commitment from the entire development team. Encourage security awareness through regular training and workshops. Establish coding standards that prioritize security, and conduct peer reviews to catch potential issues early.
Additionally, adopt a DevSecOps approach, which integrates security practices into every stage of the development lifecycle. This ensures that security is a continuous process, rather than an afterthought.
Conclusion
Writing secure code is an ongoing journey that requires vigilance, knowledge, and a proactive mindset. By adopting the principles and techniques outlined in this guide, developers can create applications that are resilient to attacks and instill confidence in users.
Secure coding is not just about protecting applications—it’s about building trust and ensuring that technology serves as a force for good. Start implementing these best practices today, and make security an integral part of your development philosophy.