Published
- 5 min read
Preventing SQL Injection Attacks in Web Applications
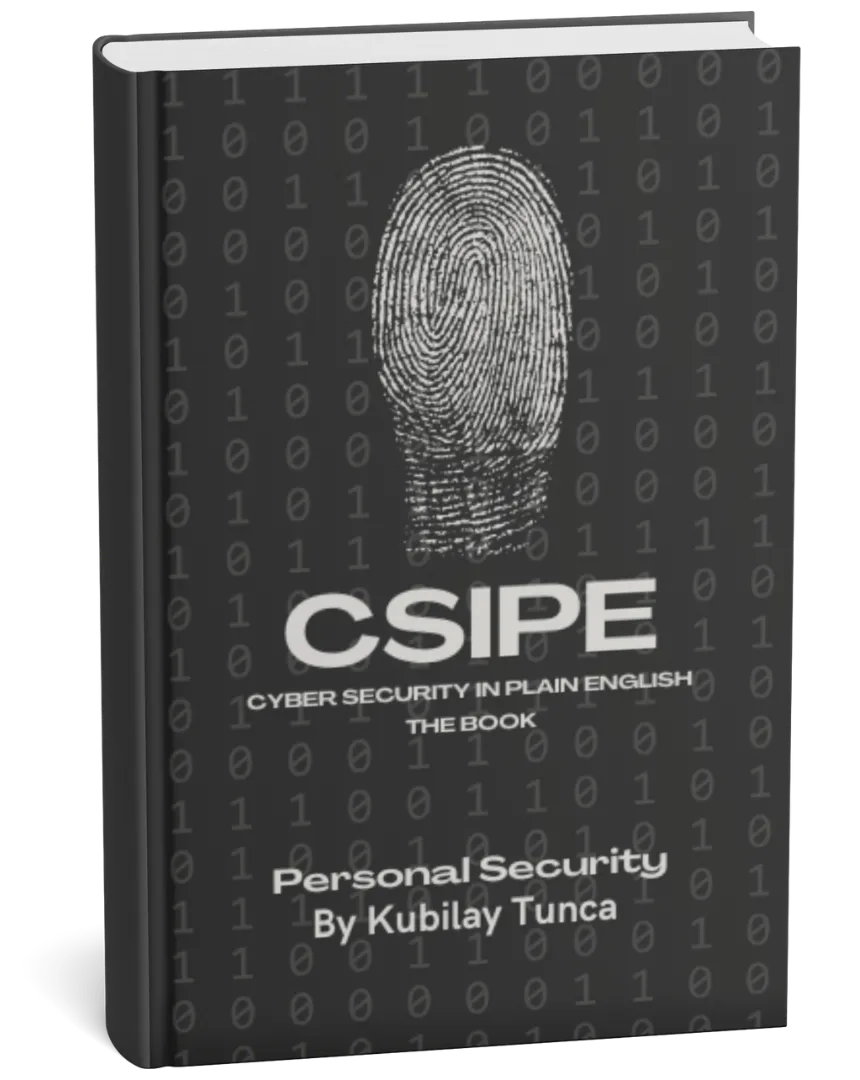
Protect Your Digital Life, Hassle-Free
Private Security in Plain English is your friendly guide to stopping common cyber threats—no tech degree required. Learn the simple, practical steps to safeguard your passwords, devices, and personal data in a language you’ll actually understand.
Buy the Ebook NowIntroduction
SQL injection attacks remain one of the most prevalent and dangerous vulnerabilities in web applications. These attacks exploit improper handling of user input in SQL queries, allowing malicious actors to manipulate databases, steal sensitive information, or even take control of servers. Despite the well-documented nature of this threat, SQL injection vulnerabilities persist, making it critical for developers to implement robust defenses.
This article delves into the mechanics of SQL injection, explores its consequences, and provides effective techniques to safeguard web applications from this pervasive security risk.
Understanding SQL Injection
SQL injection occurs when an attacker manipulates SQL queries by injecting malicious input into fields such as login forms, search boxes, or URL parameters. If the application fails to properly validate or sanitize this input, the attacker can execute unauthorized SQL commands.
How SQL Injection Works
A typical SQL injection attack follows these steps:
-
User Input: The attacker enters malicious input into a vulnerable field.
-
Query Manipulation: The malicious input is incorporated into an SQL query without proper validation or sanitization.
-
Execution: The database executes the altered query, granting the attacker access to data or control.
Example of a Vulnerable Query (PHP):
$username = $_GET['username'];
$password = $_GET['password'];
$query = "SELECT * FROM users WHERE username = '$username' AND password = '$password'";
If an attacker inputs admin' OR '1'='1
for the username, the query becomes:
SELECT * FROM users WHERE username = 'admin' OR '1'='1' AND password = 'password';
This query bypasses authentication, granting unauthorized access.
Consequences of SQL Injection
The impact of a successful SQL injection attack can be devastating, both for organizations and their users:
-
Data Theft: Attackers can retrieve sensitive information, such as user credentials, financial data, or personal details.
-
Data Manipulation: Unauthorized users can modify or delete data, leading to data corruption or loss.
-
System Compromise: SQL injection can serve as a gateway to executing commands on the underlying server.
-
Reputation Damage: Data breaches resulting from SQL injection erode user trust and damage the organization’s reputation.
-
Regulatory Penalties: Failing to protect user data may result in fines under regulations such as GDPR or PCI DSS.
Techniques to Prevent SQL Injection
Preventing SQL injection requires a multi-layered approach that incorporates secure coding practices, input validation, and robust database configuration.
1. Use Parameterized Queries
Parameterized queries, also known as prepared statements, separate query logic from user input. This ensures that user input is treated as data, not executable code.
Example (Python with SQLite):
import sqlite3
connection = sqlite3.connect("database.db")
cursor = connection.cursor()
username = input("Enter username: ")
password = input("Enter password: ")
query = "SELECT * FROM users WHERE username = ? AND password = ?"
cursor.execute(query, (username, password))
2. Implement Input Validation
Validate all user inputs to ensure they conform to expected formats. For example:
- Restrict username fields to alphanumeric characters.
- Define maximum and minimum lengths for inputs.
- Reject inputs containing special characters unless explicitly required.
3. Use Stored Procedures
Stored procedures are precompiled database queries that limit the scope for manipulation. By restricting direct SQL access, they reduce the risk of injection.
Example (MySQL):
CREATE PROCEDURE GetUser(IN username VARCHAR(50), IN password VARCHAR(50))
BEGIN
SELECT * FROM users WHERE username = username AND password = password;
END;
4. Escape Special Characters
Escaping special characters prevents them from being interpreted as part of the SQL syntax. However, this method should be a secondary measure, not a substitute for parameterized queries.
Example (PHP):
$username = mysqli_real_escape_string($connection, $_POST['username']);
$query = "SELECT * FROM users WHERE username = '$username'";
5. Use the Principle of Least Privilege
Restrict database user permissions to the minimum required for the application. For example:
- Use a read-only account for fetching data.
- Limit write and delete permissions to administrative actions.
6. Regularly Update and Patch Software
Outdated software, including database management systems, frameworks, and libraries, may contain vulnerabilities that attackers can exploit. Keep your systems up to date with the latest security patches.
7. Monitor and Log SQL Activity
Monitor database queries for unusual patterns, such as frequent failed login attempts or queries with unexpected keywords. Logging this activity can help detect and mitigate attacks.
8. Use Web Application Firewalls (WAFs)
A WAF can identify and block malicious SQL queries before they reach the database. While not a substitute for secure coding, WAFs add an additional layer of protection.
Testing for SQL Injection Vulnerabilities
Regular security testing is essential to identify and remediate vulnerabilities. Consider the following approaches:
Manual Testing
- Attempt to inject SQL commands into input fields to identify vulnerabilities.
- Use common SQL injection payloads, such as
' OR '1'='1
orUNION SELECT
.
Automated Tools
- SQLmap: A powerful tool for detecting and exploiting SQL injection vulnerabilities.
- Burp Suite: A comprehensive platform for web application security testing.
Building a Culture of Security
Preventing SQL injection is not just about implementing specific techniques—it requires fostering a culture of security within your development team. Encourage practices such as:
- Conducting regular code reviews with a focus on security.
- Providing training on secure coding practices and common vulnerabilities.
- Integrating security checks into the development lifecycle using CI/CD pipelines.
Conclusion
SQL injection is a critical threat to web applications, but it is entirely preventable with the right strategies. By adopting secure coding practices, such as parameterized queries, input validation, and least privilege principles, developers can protect their applications from this pervasive vulnerability.
Remember, security is an ongoing process. Regular testing, monitoring, and education are essential to staying ahead of evolving threats. Start implementing these techniques today to safeguard your web applications and build trust with your users.