Published
- 4 min read
Securing Microservices Architecture
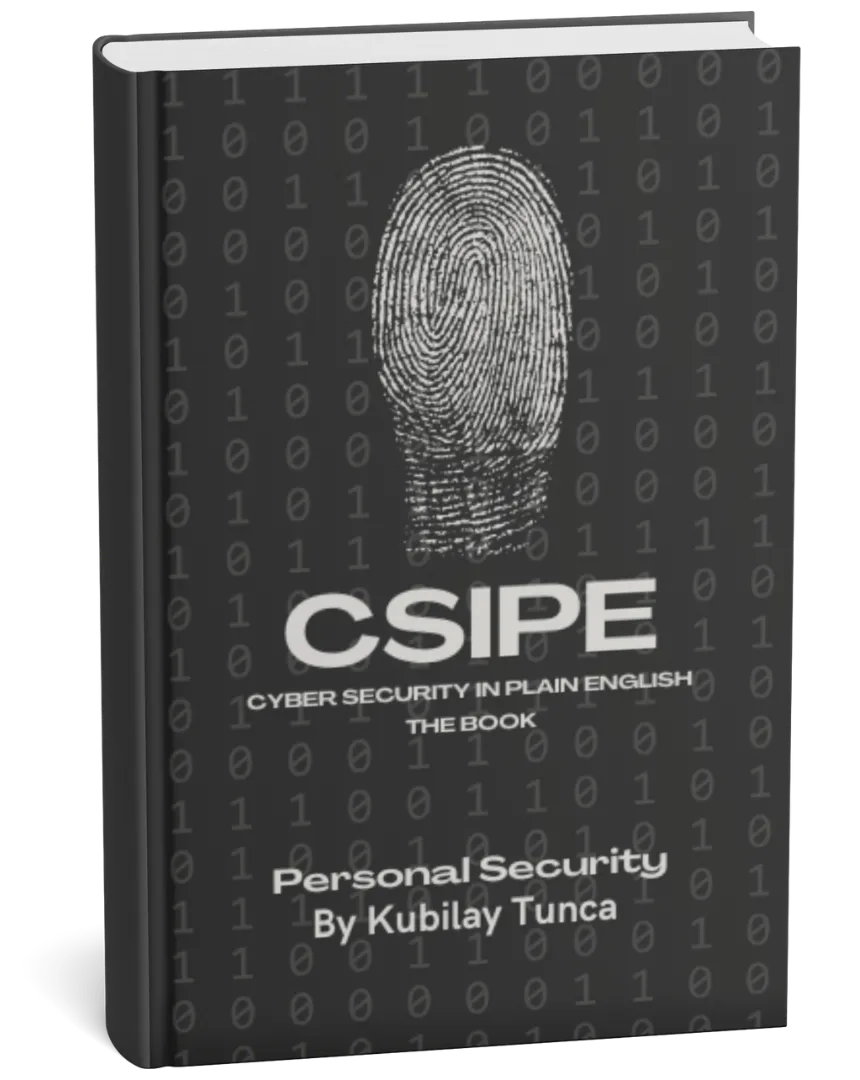
Protect Your Digital Life, Hassle-Free
Private Security in Plain English is your friendly guide to stopping common cyber threats—no tech degree required. Learn the simple, practical steps to safeguard your passwords, devices, and personal data in a language you’ll actually understand.
Buy the Ebook NowIntroduction
The microservices architecture has transformed application development by breaking down monolithic systems into smaller, independently deployable services. While this approach offers scalability and flexibility, it also introduces unique security challenges. Each service in a microservices architecture becomes a potential attack vector, necessitating robust security measures to protect inter-service communication, data, and user trust.
This guide delves into the complexities of securing microservices, highlighting best practices and strategies for developers and DevOps teams.
Why Security is Crucial in Microservices
In a microservices environment, services communicate over networks, often exposing APIs to external or internal systems. This openness, coupled with the distributed nature of microservices, increases the attack surface. Without proper security measures, vulnerabilities in one service can compromise the entire architecture.
Key Risks:
- Data Breaches:
- Sensitive data may be exposed if encryption and access controls are inadequate.
- Unauthorized Access:
- Weak authentication and authorization mechanisms can lead to compromised services.
- Service Interruption:
- Attacks on a single service can cascade, disrupting dependent services.
- Configuration Errors:
- Misconfigured environments or inadequate security settings can introduce vulnerabilities.
Core Principles of Microservices Security
1. Secure API Gateways
API gateways serve as a central entry point for external traffic, providing features such as authentication, rate limiting, and request validation. By securing the API gateway, you establish a robust first line of defense for your microservices.
Example (API Gateway Authentication):
const express = require('express')
const app = express()
app.use('/api', (req, res, next) => {
const token = req.headers.authorization
if (!token || token !== 'valid-token') {
return res.status(401).send('Unauthorized')
}
next()
})
2. Implement Strong Authentication and Authorization
Each service should authenticate and authorize requests, even if they originate from other internal services. Use token-based mechanisms, such as OAuth2 or JSON Web Tokens (JWT), for secure authentication.
Example (JWT in Node.js):
const jwt = require('jsonwebtoken')
// Verify JWT
app.use('/service', (req, res, next) => {
const token = req.headers.authorization.split(' ')[1]
jwt.verify(token, 'secretKey', (err, decoded) => {
if (err) return res.status(401).send('Unauthorized')
req.user = decoded
next()
})
})
3. Encrypt Communication
All communication between microservices should be encrypted to prevent data interception. Use TLS for securing HTTP traffic and mutual TLS (mTLS) for authenticating both client and server in inter-service communication.
Example (mTLS in Nginx):
server {
listen 443 ssl;
ssl_certificate /path/to/server.crt;
ssl_certificate_key /path/to/server.key;
ssl_client_certificate /path/to/ca.crt;
ssl_verify_client on;
location /service {
proxy_pass http://backend-service;
}
}
4. Enforce the Principle of Least Privilege
Each service should have access only to the resources it needs to perform its function. Role-based access control (RBAC) and network segmentation help limit unnecessary access.
Example (RBAC Policy in Kubernetes):
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: backend
name: backend-reader
rules:
- apiGroups: ['']
resources: ['pods']
verbs: ['get', 'list']
5. Use Secure Configuration Management
Store sensitive configurations, such as API keys and database credentials, securely using secret management tools like HashiCorp Vault, AWS Secrets Manager, or Kubernetes Secrets.
Example (Kubernetes Secret):
apiVersion: v1
kind: Secret
metadata:
name: db-credentials
type: Opaque
data:
username: YWRtaW4=
password: cGFzc3dvcmQ=
6. Implement Rate Limiting and Circuit Breakers
Prevent abuse and ensure system stability by limiting the number of requests a service can handle. Circuit breakers can help gracefully handle service failures.
Example (Rate Limiting with Express.js):
const rateLimit = require('express-rate-limit')
const limiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100 // Limit each IP to 100 requests per windowMs
})
app.use('/api/', limiter)
7. Monitor and Audit Logs
Monitor inter-service communication and maintain detailed logs for debugging and forensic analysis. Use centralized logging tools like ELK Stack or Fluentd.
Example (Nginx Access Logs):
log_format main '$remote_addr - $remote_user [$time_local] "$request" '
'$status $body_bytes_sent "$http_referer" '
'"$http_user_agent" "$http_x_forwarded_for"';
access_log /var/log/nginx/access.log main;
Challenges and Solutions in Securing Microservices
Managing Distributed Systems
Challenge: The distributed nature of microservices complicates security management. Solution: Use orchestration platforms like Kubernetes to centralize and automate security policies.
Maintaining Consistency Across Services
Challenge: Ensuring all services adhere to security standards. Solution: Implement CI/CD pipelines with integrated security checks to enforce consistent configurations.
Balancing Security with Performance
Challenge: Security measures can increase latency. Solution: Optimize encryption protocols and leverage lightweight authentication mechanisms.
Conclusion
Securing a microservices architecture is a multifaceted challenge that requires robust tools, clear strategies, and ongoing vigilance. By applying the principles outlined in this guide—such as encrypted communication, secure authentication, and centralized logging—developers can build resilient systems that protect sensitive data and maintain operational integrity.
Start integrating these practices today to safeguard your microservices and ensure the success of your applications in a competitive digital landscape.