Published
- 4 min read
Best Practices for Session Management
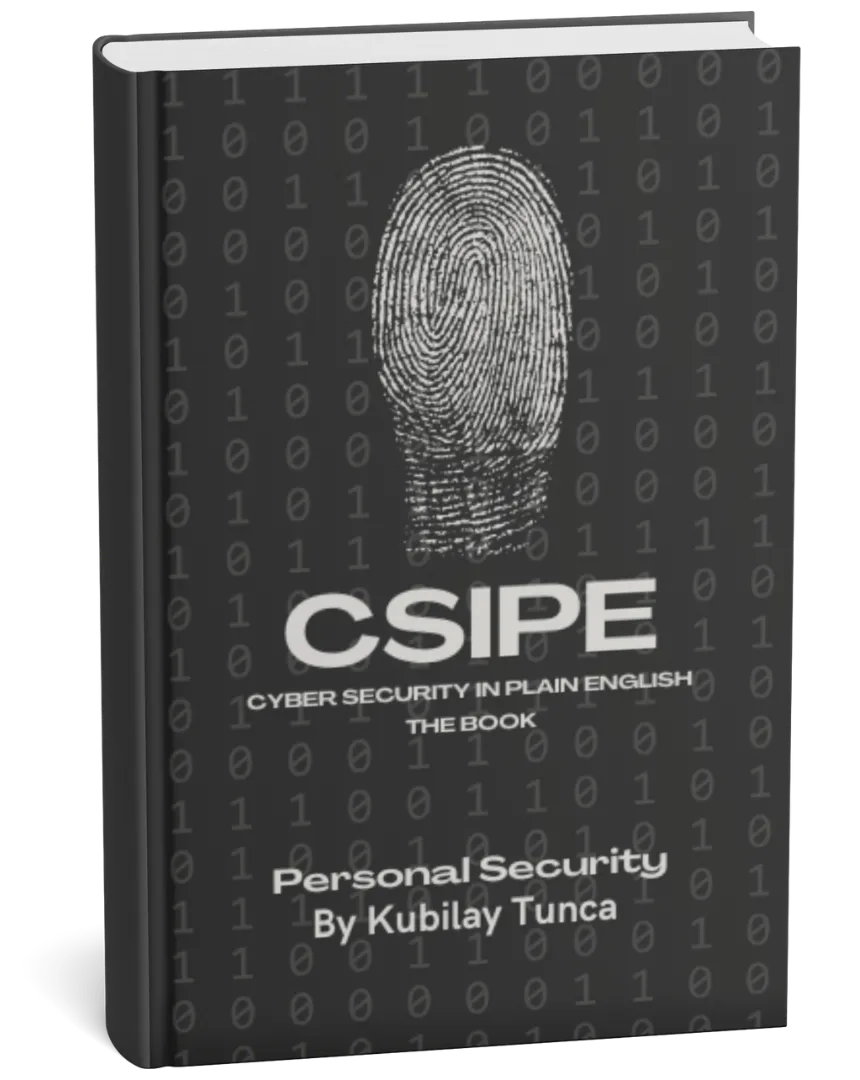
Protect Your Digital Life, Hassle-Free
Private Security in Plain English is your friendly guide to stopping common cyber threats—no tech degree required. Learn the simple, practical steps to safeguard your passwords, devices, and personal data in a language you’ll actually understand.
Buy the Ebook NowIntroduction
Session management is a cornerstone of web application security, facilitating user authentication and state maintenance across requests. While sessions enhance usability, they also present significant security challenges. Improperly implemented session management can lead to vulnerabilities like session hijacking, fixation, or exposure, putting user data and application integrity at risk.
This comprehensive guide explores the intricacies of session management, highlights common pitfalls, and outlines best practices for implementing secure session mechanisms in web applications.
Understanding Session Management
A session represents a temporary interactive information exchange between a user and a server. Sessions are critical for maintaining context in stateless HTTP protocols, enabling features like user authentication, shopping carts, and personalized dashboards.
Key Concepts in Session Management
- Session Identifiers (Session IDs):
- A unique string assigned to a user session to distinguish it from others.
- Typically stored as a cookie, query string parameter, or header.
- Session State:
- The server-side data associated with a session, including user preferences, authentication status, and application-specific details.
- Session Lifecycle:
- Includes creation, maintenance, and termination phases.
Security Risks in Session Management
1. Session Hijacking
Attackers steal active session IDs to impersonate users. This can occur via man-in-the-middle (MITM) attacks, unencrypted connections, or script injection.
2. Session Fixation
An attacker provides a user with a predefined session ID, allowing them to take control once the user logs in.
3. Cross-Site Scripting (XSS)
Malicious scripts exploit vulnerabilities to access session data stored in cookies or local storage.
4. Weak Session Expiry
Sessions that remain active indefinitely increase the risk of unauthorized access if credentials are compromised.
Best Practices for Secure Session Management
1. Use Secure Session Identifiers
Characteristics of a Secure Session ID:
- Long, random, and unique.
- Resistant to guessing and brute-force attacks.
Example (Generating Secure IDs in Node.js):
const crypto = require('crypto')
function generateSessionID() {
return crypto.randomBytes(32).toString('hex')
}
Avoid predictable session IDs:
- Do not use sequential IDs or user-specific information (e.g., username or email) as session identifiers.
2. Use HTTPS Exclusively
All session data should be transmitted over HTTPS to prevent interception by attackers. Enabling HTTPS encrypts communication between the client and server, making it difficult for malicious actors to eavesdrop.
Example (Enforcing HTTPS in Express.js):
app.use((req, res, next) => {
if (!req.secure) {
return res.redirect(`https://${req.headers.host}${req.url}`)
}
next()
})
3. Store Sessions Securely
Cookies:
- Use
HttpOnly
cookies to prevent client-side scripts from accessing session data. - Set the
Secure
flag to ensure cookies are only sent over HTTPS. - Use the
SameSite
attribute to restrict cross-site requests.
Example (Cookie Configuration in Express.js):
app.use(
require('cookie-session')({
name: 'session',
keys: ['secretKey'],
cookie: {
secure: true,
httpOnly: true,
sameSite: 'Strict'
}
})
)
4. Implement Proper Session Timeout and Expiry
Idle Timeout:
Automatically log out users after a period of inactivity to limit exposure if a session is hijacked.
Absolute Timeout:
Terminate all sessions after a fixed duration, regardless of activity.
5. Regenerate Session IDs
Regenerate session identifiers upon login or privilege escalation to prevent session fixation attacks.
Example (Session Regeneration in Express.js):
app.post('/login', (req, res) => {
req.session.regenerate((err) => {
if (err) return res.status(500).send('Error regenerating session')
req.session.user = req.body.username
res.redirect('/dashboard')
})
})
6. Secure Session Storage
Avoid storing sensitive session data on the client side. Store it server-side in memory, databases, or distributed caches like Redis.
7. Monitor and Log Session Activity
Track session usage to detect anomalies such as simultaneous logins from different IPs or geographic regions. Employ tools like Splunk or Elasticsearch for monitoring.
8. Implement Multi-Factor Authentication (MFA)
Enhance session security by requiring users to verify their identities through multiple authentication methods, such as passwords, SMS codes, or biometric data.
9. Use Content Security Policy (CSP)
Prevent XSS attacks by defining which scripts are allowed to run on your application.
Example (CSP Header in Express.js):
app.use((req, res, next) => {
res.setHeader('Content-Security-Policy', "default-src 'self'")
next()
})
Advanced Techniques for Enhanced Security
Single Sign-On (SSO)
Centralize session management across multiple applications to streamline security and usability.
Token-Based Authentication
Use access tokens instead of traditional session cookies for modern APIs and Single Page Applications (SPAs).
Challenges and Solutions in Session Management
Challenge: Balancing Security and Usability
Solution:
- Use adaptive session timeouts based on user behavior.
- Employ fingerprinting to verify devices without disrupting user experience.
Challenge: Managing Session State at Scale
Solution:
- Use distributed session stores like Redis for large-scale applications.
- Ensure session data is replicated across clusters for high availability.
Tools for Secure Session Management
1. OWASP ZAP
Detect session vulnerabilities like fixation or hijacking.
2. Redis
A high-performance in-memory database for session storage.
3. Snyk
Scan dependencies for session management vulnerabilities.
Conclusion
Secure session management is a critical pillar of web application security. By implementing best practices like secure session identifiers, HTTPS enforcement, and proper timeout policies, developers can significantly reduce the risk of session-related attacks. Coupled with robust tools and ongoing monitoring, these measures ensure user data safety and application reliability.
Start securing your sessions today to protect your users and your business in an increasingly threat-filled digital landscape.